unity 绝对路径如何转resource路径,代码实现
时间: 2024-10-05 08:01:40 浏览: 6
在Unity中,将绝对路径转换为资源路径通常是通过`AssetDatabase.GetResourcePath()`函数来完成的。这个函数会把外部文件路径转换成Unity内部可以使用的资源路径。假设你有一个外部文件的绝对路径`string absolutePath`,你可以这样做:
```csharp
using UnityEngine;
using UnityEditor;
public static string ConvertToResourcePath(string absolutePath) {
return AssetDatabase.GetResourcePath(absolutePath);
}
```
在这个例子中,`ConvertToResourcePath`函数接收一个绝对路径作为输入,然后返回对应的资源路径。如果你想要获取资源下的子文件,可以传递完整的资源路径,Unity会自动处理路径的解析。
例如,如果你有`Resources/AbsoluteFolder/SomeFile.png`,转换后的资源路径应该是`"AbsoluteFolder/SomeFile.png"`(注意没有开头的斜杠`/`)。
相关问题
unity 绝对路径转resource路径,代码实现
在Unity引擎中,当你需要将绝对路径转换为资源路径以便于内部访问时,可以使用`Resources.Load`或`AssetDatabase.GetAssetPath`等方法。这里是一个简单的代码示例:
```csharp
using UnityEngine;
using System.IO;
public static class AssetPathUtils {
public static string AbsoluteToResourcePath(string absolutePath) {
// 假设你的项目根目录是Assets,并且绝对路径是从这里开始的
string rootPath = Application.dataPath + "/..";
// 获取相对路径
string relativePath = Path.Combine(Path.GetDirectoryName(absolutePath).Replace(rootPath, ""), Path.GetFileName(absolutePath));
// 转换为Unity资源路径格式
return "Resources/" + relativePath.Replace("\\", "/");
}
}
// 使用示例
string absoluteFilePath = "Assets/Resources/myTexture.png"; // 这是一个绝对路径
string resourcePath = AssetPathUtils.AbsoluteToResourcePath(absoluteFilePath);
```
在这个例子中,我们首先获取项目的根目录(通常是`Application.dataPath`),然后计算出相对于这个根目录的相对路径。最后,我们将路径转换为Unity资源路径格式,即以"Resources/"开头。
unity 代码实现绝对路径转resource路径
在Unity中,绝对路径和Resource路径主要用于游戏资源的组织和引用。当你有一个从硬盘上直接读取的绝对路径文件,比如`"/Assets/Resources/myFile.txt"`,你需要将其转换成Unity内部可以识别的Resource路径,以便项目能够通过`Resources.Load()`函数加载。
以下是将绝对路径转换为Resource路径的基本步骤:
1. 获取相对路径:首先,你需要去掉路径的开头部分,通常是"Assets/"或"Project/",这取决于你的项目结构。如果路径是从硬盘根目录开始的,你只需保留剩余部分。
例如:
```csharp
string absolutePath = "/Assets/Resources/myFile.txt";
string relativePath = absolutePath.Substring("Assets/Resources".Length);
```
2. 使用`AssetDatabase`:然后你可以使用Unity的`AssetDatabase`类将这个相对路径转换为Resource路径。Unity会自动处理路径中的斜杠,并确保其正确格式化。
```csharp
string resourcePath = AssetDatabase.resourcePath + "/" + relativePath;
```
完整示例:
```csharp
string absolutePath = "/Assets/Resources/myFile.txt";
string relativePath = absolutePath.Substring("Assets/Resources".Length);
string assetDatabasePath = Application.dataPath;
if (!assetDatabasePath.EndsWith("/")) {
assetDatabasePath += "/";
}
string resourcePath = assetDatabasePath + relativePath;
// 现在你可以用它加载资源
object loadedObject = Resources.Load(resourcePath);
```
相关推荐
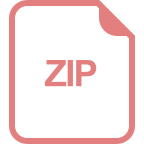
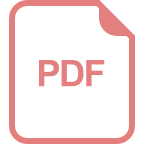
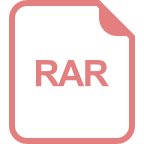

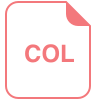
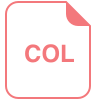









