用c语言输入某同学10门课程的成绩,找出最高分,并统计每个等级的门数
时间: 2024-11-05 11:28:11 浏览: 57
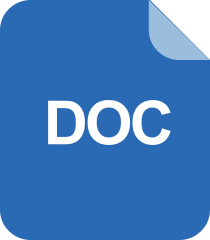
C语言输入学生成绩,计算并输出这些学生的最低分、最高分、平均分。
在C语言中,你可以使用数组和循环结构来实现这个功能。首先,创建一个数组存储学生的成绩,然后遍历数组找到最高分,同时用另一个数组或哈希表记录每个等级对应的门数。这里是一个简单的示例:
```c
#include <stdio.h>
#include <string.h>
#define MAX_SUBJECTS 10
#define GRADES { "A", "B", "C", "D", "F" }
int main() {
int scores[MAX_SUBJECTS];
char grade_counts[5] = {0}; // 初始化等级计数数组
// 输入10门课程成绩
for (int i = 0; i < MAX_SUBJECTS; ++i) {
printf("请输入第%d门课程的成绩(0-100): ", i+1);
scanf("%d", &scores[i]);
// 根据成绩范围分配等级
if (scores[i] >= 90)
grade_counts[0]++;
else if (scores[i] >= 80)
grade_counts[1]++;
else if (scores[i] >= 70)
grade_counts[2]++;
else if (scores[i] >= 60)
grade_counts[3]++;
else
grade_counts[4]++;
}
// 找到最高分
int max_score = scores[0], highest_index = 0;
for (int i = 1; i < MAX_SUBJECTS; ++i)
if (scores[i] > max_score)
max_score = scores[i], highest_index = i;
printf("最高分为:%d 分,对应课程序号:%d\n", max_score, highest_index + 1);
// 输出每个等级的门数
for (int i = 0; i < sizeof(GRADES); ++i) {
printf("%s级分数有 %d 门\n", GRADES[i], grade_counts[i]);
}
return 0;
}
```
在这个程序中,用户逐次输入10门课程的成绩,然后通过if-else条件判断计算每个等级的门数。最后,找出最高分并输出结果。
阅读全文
相关推荐
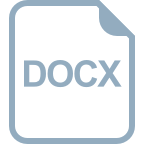

















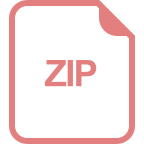