用c语言编程,输入10个学生5门课的成绩,用函数计算每个学生的平均分,每门课的平均分,找出最高分对应的学生和课程,计算平均分方差
时间: 2024-02-18 22:03:36 浏览: 39
以下是用C语言编写的程序,实现输入10个学生5门课的成绩,用函数计算每个学生的平均分,每门课的平均分,找出最高分对应的学生和课程,计算平均分方差的功能:
```c
#include <stdio.h>
#include <math.h>
#define STUDENTS 10
#define COURSES 5
void input_scores(float scores[][COURSES]);
void print_scores(float scores[][COURSES]);
void calculate_student_average(float scores[][COURSES], float student_average[]);
void calculate_course_average(float scores[][COURSES], float course_average[]);
void find_highest_score(float scores[][COURSES], int *max_student, int *max_course);
float calculate_average(float data[], int n);
float calculate_variance(float data[], int n, float average);
int main()
{
float scores[STUDENTS][COURSES];
float student_average[STUDENTS] = {0};
float course_average[COURSES] = {0};
int max_student, max_course;
float average, variance;
input_scores(scores);
printf("\nInput scores:\n");
print_scores(scores);
calculate_student_average(scores, student_average);
printf("\nStudent average scores:\n");
for(int i = 0; i < STUDENTS; i++) {
printf("Student %d: %.2f\n", i+1, student_average[i]);
}
calculate_course_average(scores, course_average);
printf("\nCourse average scores:\n");
for(int i = 0; i < COURSES; i++) {
printf("Course %d: %.2f\n", i+1, course_average[i]);
}
find_highest_score(scores, &max_student, &max_course);
printf("\nHighest score: %.2f, Student %d, Course %d\n", scores[max_student][max_course], max_student+1, max_course+1);
average = calculate_average(student_average, STUDENTS);
variance = calculate_variance(student_average, STUDENTS, average);
printf("\nStudent average score variance: %.2f\n", variance);
return 0;
}
void input_scores(float scores[][COURSES])
{
printf("Please input scores of 10 students for 5 courses:\n");
for(int i = 0; i < STUDENTS; i++) {
printf("Student %d: ", i+1);
for(int j = 0; j < COURSES; j++) {
scanf("%f", &scores[i][j]);
}
}
}
void print_scores(float scores[][COURSES])
{
for(int i = 0; i < STUDENTS; i++) {
printf("Student %d: ", i+1);
for(int j = 0; j < COURSES; j++) {
printf("%.2f ", scores[i][j]);
}
printf("\n");
}
}
void calculate_student_average(float scores[][COURSES], float student_average[])
{
for(int i = 0; i < STUDENTS; i++) {
for(int j = 0; j < COURSES; j++) {
student_average[i] += scores[i][j];
}
student_average[i] /= COURSES;
}
}
void calculate_course_average(float scores[][COURSES], float course_average[])
{
for(int i = 0; i < COURSES; i++) {
for(int j = 0; j < STUDENTS; j++) {
course_average[i] += scores[j][i];
}
course_average[i] /= STUDENTS;
}
}
void find_highest_score(float scores[][COURSES], int *max_student, int *max_course)
{
float max_score = scores[0][0];
for(int i = 0; i < STUDENTS; i++) {
for(int j = 0; j < COURSES; j++) {
if(scores[i][j] > max_score) {
max_score = scores[i][j];
*max_student = i;
*max_course = j;
}
}
}
}
float calculate_average(float data[], int n)
{
float sum = 0;
for(int i = 0; i < n; i++) {
sum += data[i];
}
return sum / n;
}
float calculate_variance(float data[], int n, float average)
{
float sum = 0;
for(int i = 0; i < n; i++) {
sum += pow(data[i] - average, 2);
}
return sum / n;
}
```
程序中定义了10个学生和5门课程,通过输入成绩、计算各种平均分、查找最高分以及计算平均分方差的函数实现不同的功能。
相关推荐
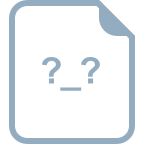
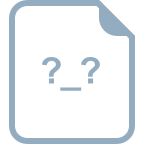
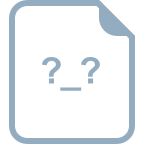














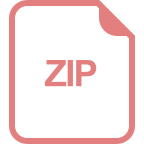