c++写一个double型二维数组连线后路径的自交叉检测函数
时间: 2024-10-12 16:16:33 浏览: 30
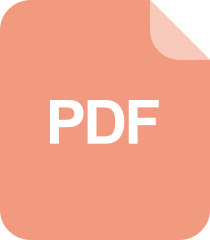
C++两个一维数组相加求和
在C++中,创建一个用于检测二维双精度数组(double类型的矩阵)中路径是否自交叉的函数可以采用广度优先搜索(BFS)算法。这里是一个简单的例子:
```cpp
#include <iostream>
#include <queue>
#include <vector>
// 定义一个二维点结构体
struct Point {
int x;
int y;
};
// 检查两点是否在同一行或同一列
bool isAdjacent(Point p1, Point p2) {
return abs(p1.x - p2.x) == 1 || abs(p1.y - p2.y) == 1;
}
// 自交检测函数
bool hasSelfIntersection(const std::vector<std::vector<double>>& grid, const Point& start, const Point& end) {
// 使用队列保存相邻的节点
std::queue<Point> q;
q.push(start);
std::vector<bool> visited(grid.size(), false);
// 初始化访问标记
visited[start.x][start.y] = true;
while (!q.empty()) {
Point current = q.front();
q.pop();
if (current == end) {
return false; // 如果到达终点,说明没有自交
}
for (int dx = -1; dx <= 1; dx++) { // 遍历四个相邻方向
int nx = current.x + dx;
int ny = current.y + (dx == 0 ? 0 : -1); // 对于垂直线,y不变
if (nx >= 0 && nx < grid.size() && ny >= 0 && ny < grid.size() && !visited[nx][ny] && grid[nx][ny] > 0) {
visited[nx][ny] = true;
q.push({nx, ny});
}
}
}
return true; // 如果没有达到终点,则表示存在自交
}
int main() {
std::vector<std::vector<double>> grid{{0, 0, 1}, {0, 1, 0}, {1, 0, 0}}; // 示例网格
Point start{0, 0};
Point end{2, 2};
bool selfIntersection = hasSelfIntersection(grid, start, end);
if (selfIntersection) {
std::cout << "The path has a self-intersection.\n";
} else {
std::cout << "The path does not have a self-intersection.\n";
}
return 0;
}
```
这个函数`hasSelfIntersection`接受一个double类型的二维数组(grid),以及起点和终点作为输入。它使用BFS遍历并检查路径是否会穿过同一格子两次。如果路径到达终点仍未发生自交,返回false;否则返回true。
阅读全文
相关推荐
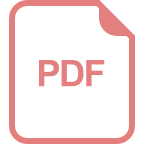
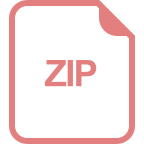
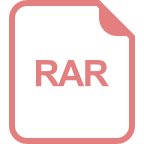
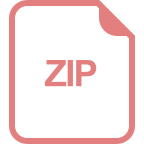





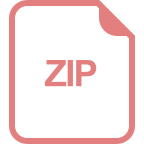
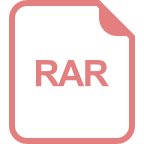
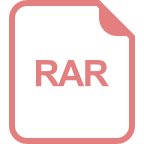