void usage(FILE *fp, int argc, char **argv)
时间: 2024-04-23 10:18:49 浏览: 11
void usage(FILE *fp, int argc, char **argv)是一个函数的声明,用于在程序中显示用法信息。它接受三个参数:一个指向FILE类型的指针fp,一个整型参数argc,以及一个指向字符指针的指针argv。
该函数通常在程序运行时,当用户输入了错误的命令行参数或者需要查看程序的使用方法时被调用。它的作用是向用户展示程序的正确使用方式和可接受的命令行参数。
函数体内部的具体实现可以根据具体需求来编写,但通常会使用fprintf函数将使用方法输出到指定的文件流fp中。argc和argv参数可以用来获取用户输入的命令行参数,以便在使用方法中进行展示或者根据不同的参数执行不同的逻辑。
相关问题
4.RLE压缩解压算法 涉及知识点:文件读写、位操作、内存管理、结构体定义、RLE算法、命令行参数 要求: 编写一个程序,可以在命令行输入参数,完成指定文件的压缩解压 命令行参数如下 rle file1 –c(-d) file2 第一个参数为可执行程序名称,第二个参数为原始文件名,第三个参数为压缩或解压缩选项,第四个参数为新文件名
以下是一个简单的RLE压缩解压程序的示例代码,使用C语言编写:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LEN 256
// 结构体定义,用于存储RLE编码的数据
typedef struct RLEData {
unsigned char value;
int count;
} RLEData;
// 函数声明
int compressRLE(char *inputFile, char *outputFile);
int decompressRLE(char *inputFile, char *outputFile);
void writeRLEData(FILE *fp, RLEData *data);
void printUsage();
int main(int argc, char *argv[]) {
char *inputFile, *outputFile;
int option;
// 检查命令行参数数量
if (argc != 5) {
printUsage();
return 0;
}
// 解析命令行参数
inputFile = argv[2];
option = argv[3][1];
outputFile = argv[4];
// 根据选项进行压缩或解压
switch (option) {
case 'c':
if (compressRLE(inputFile, outputFile))
printf("Compression completed.\n");
else
printf("Compression failed.\n");
break;
case 'd':
if (decompressRLE(inputFile, outputFile))
printf("Decompression completed.\n");
else
printf("Decompression failed.\n");
break;
default:
printUsage();
break;
}
return 0;
}
// RLE压缩函数
int compressRLE(char *inputFile, char *outputFile) {
FILE *fpIn, *fpOut;
unsigned char currChar, prevChar;
int count = 0;
RLEData data;
data.count = 0;
// 打开输入文件和输出文件
fpIn = fopen(inputFile, "rb");
if (fpIn == NULL) {
printf("Failed to open input file.\n");
return 0;
}
fpOut = fopen(outputFile, "wb");
if (fpOut == NULL) {
printf("Failed to create output file.\n");
fclose(fpIn);
return 0;
}
// 读取文件内容并进行RLE编码
prevChar = fgetc(fpIn);
while (!feof(fpIn)) {
currChar = fgetc(fpIn);
if (currChar == prevChar) {
count++;
} else {
data.value = prevChar;
data.count = count + 1;
writeRLEData(fpOut, &data);
prevChar = currChar;
count = 0;
}
}
// 处理最后一个字符
data.value = prevChar;
data.count = count + 1;
writeRLEData(fpOut, &data);
// 关闭文件
fclose(fpIn);
fclose(fpOut);
return 1;
}
// RLE解压函数
int decompressRLE(char *inputFile, char *outputFile) {
FILE *fpIn, *fpOut;
unsigned char currChar;
int count;
RLEData data;
// 打开输入文件和输出文件
fpIn = fopen(inputFile, "rb");
if (fpIn == NULL) {
printf("Failed to open input file.\n");
return 0;
}
fpOut = fopen(outputFile, "wb");
if (fpOut == NULL) {
printf("Failed to create output file.\n");
fclose(fpIn);
return 0;
}
// 读取文件内容并进行RLE解码
while (!feof(fpIn)) {
fread(&data, sizeof(RLEData), 1, fpIn);
currChar = data.value;
count = data.count;
while (count-- > 0) {
fputc(currChar, fpOut);
}
}
// 关闭文件
fclose(fpIn);
fclose(fpOut);
return 1;
}
// 写入RLE编码的数据到文件中
void writeRLEData(FILE *fp, RLEData *data) {
fwrite(data, sizeof(RLEData), 1, fp);
}
// 打印程序用法信息
void printUsage() {
printf("Usage: rle inputFile -c(-d) outputFile\n");
printf("Options:\n");
printf(" -c: compress file\n");
printf(" -d: decompress file\n");
}
```
该程序支持命令行参数,可以对指定文件进行RLE压缩或解压缩。使用方法如下:
- 压缩文件:`rle file1 -c file2`
- 解压缩文件:`rle file2 -d file1`
其中,`file1`为原始文件名,`file2`为新文件名。注意,该程序只能处理单个文件,无法处理目录。
根据以下伪代码框架,写一c语言程序//伪代码框架,仅供参考 #include <stdio.h> #include <stdlib.h> #include <string.h> // 定义单词结构体 struct Word { char english[20]; // 英语单词 char chinese[50]; // 中文解释 // 可以根据需要定义其他成员变量 }; // 读取单词列表文件,返回单词数量 int read_word_file(const char* file_name, struct Word words[], int max_words) { // 打开文件 // 如果文件打开失败,则返回错误 // 否则,读取文件内容 // 将每一行的英语单词和中文解释分别存储到 words 数组中的对应元素 // 如果已经存储的单词数量超过了 max_words,则停止读取 // 返回实际存储的单词数量 return 0; } // 随机选择一个单词,并提示用户输入该单词的英文 void test_word(const struct Word words[], int num_words) { // 随机选择一个单词 // 输出中文提示 // 读取用户输入的英文单词 // 比较用户输入的单词和正确答案,输出提示信息 } // 运行单词测试程序,返回成功背诵的单词数量 int run_word_test(const struct Word words[], int num_words) { // 定义变量:成功背诵的单词数量、开始测试时间、结束测试时间... // 循环测试单词,直到用户中途退出 // 在每次测试完成后,输出本次测试的结果(背诵时间、正确率等) // 返回成功背诵的单词数量 return 0; }
以下是根据伪代码框架编写的C语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#define MAX_WORD_LENGTH 20
#define MAX_DEFINITION_LENGTH 50
#define MAX_WORDS 1000
struct Word {
char english[MAX_WORD_LENGTH];
char chinese[MAX_DEFINITION_LENGTH];
};
int read_word_file(const char* file_name, struct Word words[], int max_words) {
FILE* fp = fopen(file_name, "r");
if (fp == NULL) {
printf("Failed to open file: %s\n", file_name);
return -1;
}
int count = 0;
char line[MAX_WORD_LENGTH + MAX_DEFINITION_LENGTH + 2];
while (fgets(line, sizeof(line), fp) != NULL && count < max_words) {
char* p = strchr(line, '\n');
if (p != NULL) {
*p = '\0';
}
p = strchr(line, '\t');
if (p == NULL) {
continue;
}
*p = '\0';
strncpy(words[count].english, line, MAX_WORD_LENGTH);
strncpy(words[count].chinese, p + 1, MAX_DEFINITION_LENGTH);
count++;
}
fclose(fp);
return count;
}
void test_word(const struct Word words[], int num_words) {
if (num_words <= 0) {
printf("No words to test.\n");
return;
}
srand(time(NULL));
int index = rand() % num_words;
const char* chinese = words[index].chinese;
printf("Please enter the English word for \"%s\": ", chinese);
char english[MAX_WORD_LENGTH + 1];
if (fgets(english, sizeof(english), stdin) == NULL) {
return;
}
char* p = strchr(english, '\n');
if (p != NULL) {
*p = '\0';
}
if (strcmp(english, words[index].english) == 0) {
printf("Correct!\n");
} else {
printf("Wrong! The correct answer is \"%s\".\n", words[index].english);
}
}
int run_word_test(const struct Word words[], int num_words) {
if (num_words <= 0) {
printf("No words to test.\n");
return 0;
}
int correct_count = 0;
time_t start_time, end_time;
double elapsed_time;
double accuracy;
int test_count = 0;
printf("Press Enter to start the test. Type \"quit\" to exit.\n");
if (fgets(NULL, 0, stdin) == NULL) {
return 0;
}
start_time = time(NULL);
while (1) {
test_word(words, num_words);
test_count++;
printf("Press Enter to continue or type \"quit\" to exit.\n");
if (fgets(NULL, 0, stdin) == NULL) {
return correct_count;
}
if (strcmp("quit\n", stdin) == 0) {
return correct_count;
}
end_time = time(NULL);
elapsed_time = difftime(end_time, start_time);
accuracy = (double)correct_count / (double)test_count * 100.0;
printf("Elapsed time: %.0f seconds, accuracy: %.0f%%.\n", elapsed_time, accuracy);
}
return correct_count;
}
int main(int argc, char* argv[]) {
if (argc != 2) {
printf("Usage: %s <word_file>\n", argv[0]);
return 1;
}
struct Word words[MAX_WORDS];
int num_words = read_word_file(argv[1], words, MAX_WORDS);
if (num_words <= 0) {
return 1;
}
int correct_count = run_word_test(words, num_words);
printf("Congratulations! You have successfully memorized %d words.\n", correct_count);
return 0;
}
```
这个程序可以从一个单词列表文件中读取单词和中文解释,然后随机选择一个单词并提示用户输入该单词的英文,最后输出测试结果(包括背诵时间和正确率等)。用户可以在测试过程中随时退出,退出后程序会输出已经成功背诵的单词数量。
相关推荐
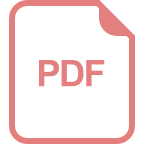
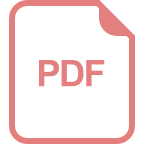
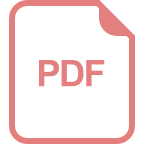












