设计一个算法,使用 C 语言实现:使用结构体和指针存储线性表结构,实现线性表的以 下操作:求链表节点总数、添加新节点、输出所有节点、任意位置插入新节点、删除节 点、查找节点。
时间: 2024-10-09 18:04:42 浏览: 45
在C语言中,我们可以设计一个简单的单向链表结构来实现上述操作。首先,我们创建一个`Node`结构体表示链表的每个元素,并包含一个数据域和指向下一个节点的指针。然后,我们需要定义一个全局的链表头指针`head`。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data; // 节点数据
struct Node* next; // 指向下一个节点的指针
} Node;
// 初始化链表为空
Node* createList() {
return NULL;
}
// 添加新节点
void insertNode(Node** head, int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = *head;
*head = newNode;
}
// 计算链表节点总数
int countNodes(Node* head) {
int count = 0;
while (head != NULL) {
count++;
head = head->next;
}
return count;
}
// 输出所有节点
void printList(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
// 在指定位置插入节点
void insertAtPosition(Node** head, int pos, int data) {
if (pos <= 0) {
printf("Invalid position.\n");
return;
}
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = *head;
Node* prev = NULL;
for (int i = 0; i < pos - 1 && head != NULL; i++) {
prev = head;
head = head->next;
}
if (prev == NULL) {
printf("Out of range.\n");
return;
}
newNode->next = head;
prev->next = newNode;
}
// 删除节点
void deleteNode(Node** head, int key) {
if (*head == NULL) {
printf("List is empty.\n");
return;
}
Node* temp = *head;
Node* prev = NULL;
if (temp->data == key) {
*head = temp->next;
free(temp);
return;
}
while (temp != NULL && temp->data != key) {
prev = temp;
temp = temp->next;
}
if (temp == NULL) {
printf("Element not found.\n");
return;
}
prev->next = temp->next;
free(temp);
}
// 查找节点
Node* findNode(Node* head, int key) {
Node* temp = head;
while (temp != NULL) {
if (temp->data == key) {
return temp;
}
temp = temp->next;
}
return NULL;
}
int main() {
Node* listHead = createList();
// 使用上述函数进行操作...
return 0;
}
```
阅读全文
相关推荐
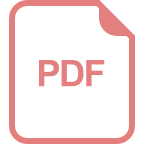
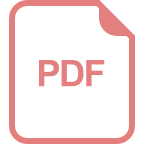
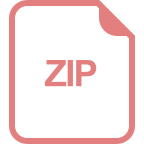
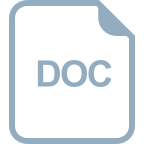
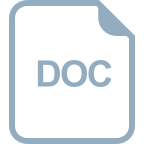
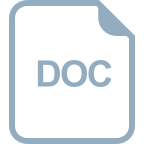
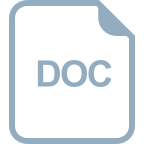
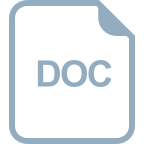
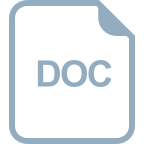
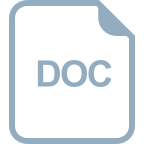
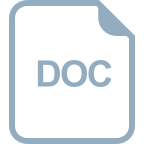
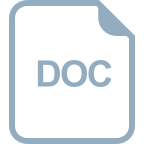
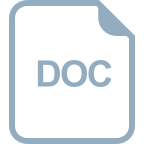
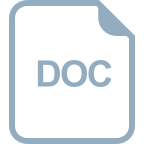



