设计一个算法,使用 C 语言实现:使用结构体和指针存储线性表结构,实现线性表的以 下操作:求链表节点总数、添加新节点、输出所有节点、任意位置插入新节点、删除节 点、查找节点。
时间: 2024-09-25 15:10:44 浏览: 39
为了设计一个 C 语言实现的线性表(也称为链表),我们可以创建一个名为 `ListNode` 的结构体来表示每个节点,包含一个数据元素和指向下一个节点的指针。这里是一个简单的实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct ListNode {
int data;
struct ListNode* next;
} ListNode;
// 初始化一个空链表
ListNode* createList() {
return (ListNode*)malloc(sizeof(ListNode));
}
// 求链表节点总数
int countNodes(ListNode* head) {
int count = 0;
ListNode* current = head;
while (current != NULL) {
count++;
current = current->next;
}
return count;
}
// 添加新节点
void addNode(ListNode** head, int value) {
ListNode* newNode = createList();
newNode->data = value;
newNode->next = *head;
*head = newNode;
}
// 输出所有节点
void printList(ListNode* head) {
printf("Nodes in the list: ");
ListNode* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
// 在任意位置插入新节点
void insertNode(ListNode** head, int position, int value) {
if (position <= 0) {
printf("Invalid position.\n");
return;
}
ListNode* newNode = createList();
newNode->data = value;
newNode->next = *head;
ListNode* previous = NULL;
ListNode* current = *head;
for (int i = 0; i < position - 1 && current != NULL; i++) {
previous = current;
current = current->next;
}
if (previous == NULL) {
newNode->next = current;
} else {
previous->next = newNode;
}
}
// 删除节点
void deleteNode(ListNode** head, int position) {
if (*head == NULL || position <= 0) {
printf("Invalid operation or empty list.\n");
return;
}
if (position == 1) { // 如果要删除头节点
ListNode* temp = *head;
*head = (*head)->next;
free(temp);
} else {
ListNode* current = *head;
ListNode* previous = NULL;
for (int i = 1; i < position && current != NULL; i++) {
previous = current;
current = current->next;
}
if (current == NULL) {
printf("Node not found.\n");
} else {
previous->next = current->next;
free(current);
}
}
}
// 查找节点
ListNode* searchNode(ListNode* head, int value) {
ListNode* current = head;
while (current != NULL) {
if (current->data == value) {
return current;
}
current = current->next;
}
return NULL; // 节点未找到
}
// 主函数演示如何使用这些操作
int main() {
ListNode* head = createList();
// 使用示例
addNode(&head, 1);
addNode(&head, 2);
addNode(&head, 3);
printList(head);
insertNode(&head, 2, 4); // 在索引2处插入值4
printList(head);
ListNode* foundNode = searchNode(head, 2); // 查找值为2的节点
if (foundNode) {
printf("Found node with value 2 at position %d\n", findNodePosition(foundNode));
} else {
printf("Value 2 not found.\n");
}
deleteNode(&head, 1); // 删除索引1的节点
return 0;
}
```
这个程序包含了你需要的所有操作,包括初始化链表、增加节点、遍历并打印节点、在指定位置插入节点、删除节点以及查找节点。记得在使用之前包含适当的数据初始化。
阅读全文
相关推荐
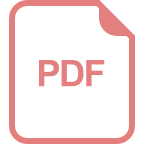
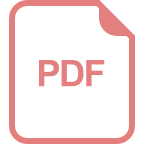
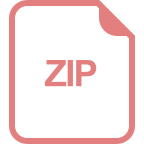
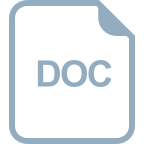
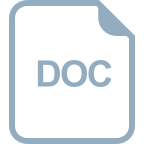
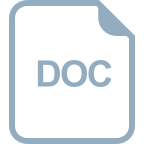
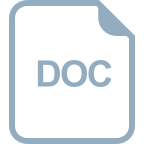
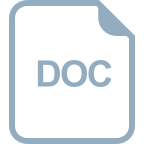
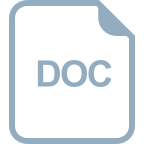
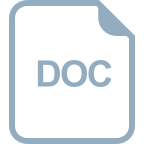
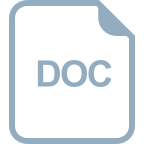
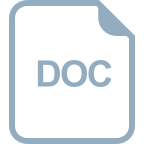
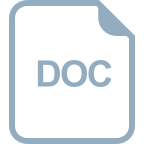
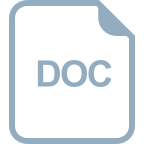



