设计一个用winform可以实现的小游戏
时间: 2024-05-04 19:20:57 浏览: 13
以下是一个简单的用 Winform 设计的小游戏,名为 "打地鼠"。
游戏规则:
- 布置在窗体上的地洞会随机出现地鼠。
- 玩家需要在规定时间内,点击出现的地鼠,以得分。
- 玩家可以选择游戏时间和难度。
实现步骤:
1. 创建一个 Winform 应用程序项目,在窗体上添加一个 PictureBox 控件用于显示地鼠图片,一个 Label 控件用于显示得分,一个 Timer 控件用于控制游戏时间。
2. 创建一个地鼠类,包含地鼠图片和位置信息等属性,以及被点击时增加分数的方法。
3. 在窗体的 Load 事件中,初始化游戏设置,如游戏时间、难度等。
4. 在 Timer 的 Tick 事件中,随机生成地洞和地鼠,并将地鼠对象添加到 PictureBox 中。
5. 在 PictureBox 的 Click 事件中,判断是否点击到地鼠,并调用地鼠的被点击方法。
6. 在游戏结束时,显示得分并提示是否重新开始游戏。
代码示例:
```csharp
public partial class Form1 : Form
{
private int score;
private int gameTime;
private int difficulty;
private Random random;
private List<Mole> moles;
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
// 初始化游戏设置
score = 0;
gameTime = 60;
difficulty = 5;
random = new Random();
moles = new List<Mole>();
timer1.Interval = difficulty * 1000;
// 显示游戏设置
lblScore.Text = $"得分:{score}";
lblTime.Text = $"时间:{gameTime}";
lblDifficulty.Text = $"难度:{difficulty}";
// 启动游戏时间计时器
timer2.Interval = 1000;
timer2.Start();
}
private void timer1_Tick(object sender, EventArgs e)
{
// 随机生成地洞和地鼠
int moleIndex = random.Next(9);
Mole mole = new Mole(moleIndex);
moles.Add(mole);
PictureBox picBox = (PictureBox)Controls.Find($"picBox{moleIndex}", true)[0];
picBox.Image = mole.Image;
picBox.Tag = mole;
}
private void picBox_Click(object sender, EventArgs e)
{
// 点击地鼠
PictureBox picBox = (PictureBox)sender;
Mole mole = (Mole)picBox.Tag;
if (mole != null)
{
score += mole.Click();
lblScore.Text = $"得分:{score}";
}
}
private void timer2_Tick(object sender, EventArgs e)
{
// 更新游戏时间
gameTime--;
lblTime.Text = $"时间:{gameTime}";
// 游戏结束
if (gameTime == 0)
{
timer1.Stop();
timer2.Stop();
MessageBox.Show($"游戏结束!得分:{score}");
if (MessageBox.Show("是否重新开始游戏?", "提示", MessageBoxButtons.YesNo) == DialogResult.Yes)
{
// 重新开始游戏
score = 0;
gameTime = 60;
difficulty = 5;
moles.Clear();
lblScore.Text = $"得分:{score}";
lblTime.Text = $"时间:{gameTime}";
lblDifficulty.Text = $"难度:{difficulty}";
timer1.Interval = difficulty * 1000;
timer1.Start();
timer2.Start();
}
else
{
// 退出游戏
Application.Exit();
}
}
}
}
public class Mole
{
private static Image[] images =
{
Properties.Resources.Mole1,
Properties.Resources.Mole2,
Properties.Resources.Mole3,
Properties.Resources.Mole4,
Properties.Resources.Mole5,
Properties.Resources.Mole6,
Properties.Resources.Mole7,
Properties.Resources.Mole8,
Properties.Resources.Mole9,
};
public Image Image { get; private set; }
public int Score { get; private set; }
public bool IsAlive { get; set; }
public int Index { get; private set; }
public Mole(int index)
{
Image = images[index];
Score = index + 1;
IsAlive = true;
Index = index;
}
public int Click()
{
if (IsAlive)
{
IsAlive = false;
return Score;
}
else
{
return 0;
}
}
}
```
相关推荐
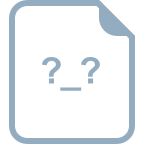
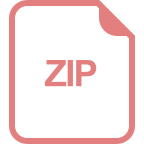
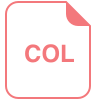













