pthread 通过 tid判断线程是否运行
时间: 2023-02-09 09:06:18 浏览: 98
pthread_t 类型是线程标识符, 可以通过 pthread_equal 函数来判断两个线程标识符是否代表同一个线程:
```
int pthread_equal(pthread_t tid1, pthread_t tid2);
```
如果 tid1 和 tid2 代表同一个线程, pthread_equal 函数会返回非零值, 否则返回 0。
但是, 如果你想要判断线程是否正在运行, 你需要使用其他方法, 比如设置一个线程标志变量, 并在线程的执行函数中进行修改, 外部可以读取这个线程标志变量来判断线程是否正在运行。
相关问题
pthread_create 创建线程 举个例子
pthread_create函数是用来创建一个新的线程的,它的原型如下:
```
int pthread_create(pthread_t *restrict tidp, const pthread_attr_t *restrict attr, void *(*start_rtn)(void*), void *restrict arg);
```
其中,tidp是指向线程ID的指针,attr是指向线程属性的指针,start_rtn是指向线程函数的指针,arg是传递给线程函数的参数。下面是一个使用pthread_create函数创建线程的例子:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
void *thread_func(void *arg)
{
int i;
for (i = 0; i < 10; i++) {
printf("Thread %d: %d\n", *(int *)arg, i);
}
return NULL;
}
int main()
{
pthread_t tid1, tid2;
int arg1 = 1, arg2 = 2;
pthread_create(&tid1, NULL, thread_func, &arg1);
pthread_create(&tid2, NULL, thread_func, &arg2);
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
return 0;
}
```
在这个例子中,我们定义了一个线程函数thread_func,它接受一个参数arg,然后在主函数中使用pthread_create函数创建了两个线程,并将arg1和arg2作为参数传递给线程函数。最后,我们使用pthread_join函数等待两个线程结束。
pthread如何获取线程标识符
pthread库中的线程标识符是一个pthread_t类型的变量,可以通过pthread_self()函数获取当前线程的标识符,例如:
```
#include <pthread.h>
#include <stdio.h>
void* thread_func(void* arg) {
pthread_t tid = pthread_self();
printf("Thread ID is %lu\n", tid);
return NULL;
}
int main() {
pthread_t tid;
pthread_create(&tid, NULL, thread_func, NULL);
pthread_join(tid, NULL);
return 0;
}
```
在上述代码中,pthread_create()函数创建了一个新线程,该线程的标识符存储在tid变量中。在线程函数中,我们通过pthread_self()获取当前线程的标识符,并打印输出。最后,我们使用pthread_join()等待线程执行结束。
相关推荐
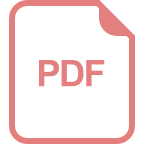












