使用pthread库编写线程栅栏测试程序
时间: 2023-08-01 15:11:09 浏览: 107
线程栅栏是一种同步机制,用于确保所有线程在达到某个点之前都被阻塞,直到所有线程都到达该点后才能继续执行。下面是一个使用pthread库编写的线程栅栏测试程序:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define NUM_THREADS 5
#define BARRIER_COUNT 5
int count = 0;
pthread_mutex_t count_mutex;
pthread_cond_t count_threshold_cv;
void *thread_func(void *thread_id)
{
int i, j;
long tid;
tid = (long)thread_id;
for (i = 0; i < BARRIER_COUNT; i++) {
pthread_mutex_lock(&count_mutex);
count++;
if (count == NUM_THREADS) {
printf("Thread %ld: Barrier reached.\n", tid);
pthread_cond_broadcast(&count_threshold_cv);
} else {
printf("Thread %ld: Waiting at barrier.\n", tid);
pthread_cond_wait(&count_threshold_cv, &count_mutex);
}
pthread_mutex_unlock(&count_mutex);
}
pthread_exit(NULL);
}
int main()
{
int i;
long thread_ids[NUM_THREADS];
pthread_t threads[NUM_THREADS];
pthread_mutex_init(&count_mutex, NULL);
pthread_cond_init(&count_threshold_cv, NULL);
for (i = 0; i < NUM_THREADS; i++) {
thread_ids[i] = i;
pthread_create(&threads[i], NULL, thread_func, (void *)&thread_ids[i]);
}
for (i = 0; i < NUM_THREADS; i++) {
pthread_join(threads[i], NULL);
}
pthread_mutex_destroy(&count_mutex);
pthread_cond_destroy(&count_threshold_cv);
return 0;
}
```
该程序创建了5个线程,每个线程都会执行5次循环。在每次循环中,线程都会增加计数器的值,然后等待其他线程到达同一个点。当所有线程都到达该点时,一个线程会打印一条消息,然后广播一个条件变量以释放所有线程。
这个程序展示了线程栅栏的基本思想和实现方式。通过使用互斥锁和条件变量,我们可以确保所有线程在到达某个点之前都被阻塞,直到所有线程都到达该点后才能继续执行。
阅读全文
相关推荐









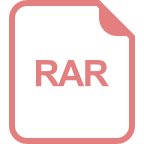

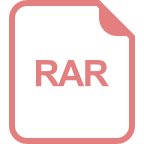




