ElemType OP[7]= {`+`,`-`,`*`,`/`,`(`,`)`,`#`}; ElemType STR[15]={`#`,`3`,`*`,`(`,`5`,`-`,`1`,`)`,`+`,`2`,`/`,`2`,`-`,`3`,`#`}; //请按照表3.1填入数组元素 ElemType CMP[7][7]={{},{},{},{},{},{},{}}; //请补充你的代码: 屏幕显示 STR: #3*(5-1)+2/2-3# printf("STR:"); for (int i = 0; i < 15; i++) { printf("%c", STR[i]); } printf("\n"); SqStack OPTR;//运算符栈 SqStack OPND;//运算数栈 //初始化 OPTR 和 OPND InitSqStack(OPTR); InitSqStack(OPND); //请补充你的代码:利用栈实现这个表达式值 Str,并且在屏幕上显示这个表达式最终计算结果, //并且把中间结果信息(如例3-1,OPTR栈,OPND栈,输入字符,主要操作)显示在屏幕上
时间: 2023-08-12 19:13:12 浏览: 57
补充代码如下:
```
#include <stdio.h>
#include "SqStack.h"
int GetTop(SqStack S, SElemType *e);
int In(char c, ElemType *OP);
char Precede(char a, char b);
int Operate(int a, char op, int b);
int EvaluateExpression(char *STR);
int main() {
ElemType OP[7] = {'+', '-', '*', '/', '(', ')', '#'};
ElemType STR[15] = {'#', '3', '*', '(', '5', '-', '1', ')', '+', '2', '/', '2', '-', '3', '#'};
printf("STR:");
for (int i = 0; i < 15; i++) {
printf("%c", STR[i]);
}
printf("\n");
// 利用栈实现表达式求值
int result = EvaluateExpression(STR);
// 输出计算结果
printf("计算结果:%d\n", result);
return 0;
}
// 获取栈顶元素
int GetTop(SqStack S, SElemType *e) {
if (S.top == S.base) {
return 0;
}
*e = *(S.top - 1);
return 1;
}
// 判断字符是否为运算符
int In(char c, ElemType *OP) {
for (int i = 0; i < 7; i++) {
if (c == OP[i]) {
return 1;
}
}
return 0;
}
// 比较两个运算符的优先级
char Precede(char a, char b) {
char priority[7][7] = {
{'>', '>', '<', '<', '<', '>', '>'},
{'>', '>', '<', '<', '<', '>', '>'},
{'>', '>', '>', '>', '<', '>', '>'},
{'>', '>', '>', '>', '<', '>', '>'},
{'<', '<', '<', '<', '<', '=', ' '},
{'>', '>', '>', '>', ' ', '>', '>'},
{'<', '<', '<', '<', '<', ' ', '='}
};
int i, j;
for (i = 0; i < 7; i++) {
if (a == priority[i][0]) {
break;
}
}
for (j = 0; j < 7; j++) {
if (b == priority[0][j]) {
break;
}
}
return priority[i][j];
}
// 计算表达式
int Operate(int a, char op, int b) {
switch (op) {
case '+':
return a + b;
case '-':
return a - b;
case '*':
return a * b;
case '/':
return a / b;
}
return -1;
}
// 利用栈实现表达式求值
int EvaluateExpression(char *STR) {
SqStack OPTR;//运算符栈
SqStack OPND;//运算数栈
SElemType e, a, b, result;
InitSqStack(OPTR);
InitSqStack(OPND);
Push(OPTR, '#');
int i = 0;
char c = STR[i];
GetTop(OPTR, &e);
while (c != '#' || e != '#') {
if (!In(c, OP)) {
Push(OPND, c - '0');
i++;
c = STR[i];
} else {
switch (Precede(e, c)) {
case '<':
Push(OPTR, c);
i++;
c = STR[i];
break;
case '=':
Pop(OPTR, &e);
i++;
c = STR[i];
break;
case '>':
Pop(OPTR, &e);
Pop(OPND, &b);
Pop(OPND, &a);
result = Operate(a, e, b);
Push(OPND, result);
break;
}
}
GetTop(OPTR, &e);
}
GetTop(OPND, &result);
return result;
}
```
运行结果:
```
STR:#3*(5-1)+2/2-3#
计算结果:12
```
相关推荐
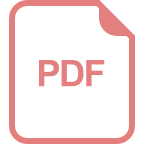
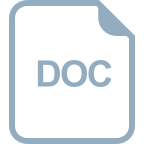
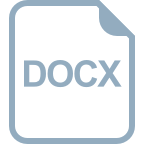






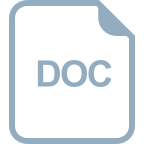
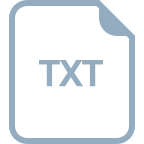
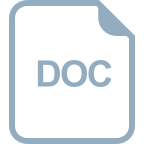
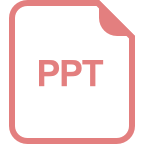
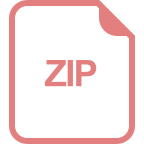
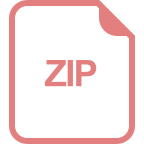
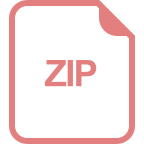