使用OpenGL中的函数绘制几*几个控制点(建议4*4以上)的一个Bezier曲面
时间: 2024-01-21 18:16:15 浏览: 93
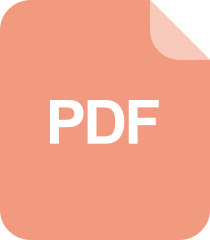
在VC++中使用OpenGL绘制典型曲面
以下是使用OpenGL绘制4x4个控制点的Bezier曲面的示例代码:
```c++
#include <GL/glut.h>
// 控制点坐标
GLfloat ctrlPoints[4][4][3] = {
{{-1.5, -1.5, 4.0}, {-0.5, -1.5, 2.0}, {0.5, -1.5, -1.0}, {1.5, -1.5, 2.0}},
{{-1.5, -0.5, 1.0}, {-0.5, -0.5, 3.0}, {0.5, -0.5, 0.0}, {1.5, -0.5, -1.0}},
{{-1.5, 0.5, 4.0}, {-0.5, 0.5, 0.0}, {0.5, 0.5, 3.0}, {1.5, 0.5, 4.0}},
{{-1.5, 1.5, -2.0}, {-0.5, 1.5, -2.0}, {0.5, 1.5, 0.0}, {1.5, 1.5, -1.0}}
};
void init()
{
glClearColor(0.0, 0.0, 0.0, 1.0);
glEnable(GL_DEPTH_TEST);
glShadeModel(GL_FLAT);
}
void display()
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glColor3f(1.0, 1.0, 1.0);
glPushMatrix();
glRotatef(45.0, 1.0, 1.0, 1.0);
for (int i = 0; i < 30; i++) {
glMapGrid2f(30, 0.0, 1.0, 30, 0.0, 1.0);
glEvalMesh2(GL_FILL, 0, 30, 0, 30);
}
glPopMatrix();
glutSwapBuffers();
}
void reshape(int w, int h)
{
glViewport(0, 0, (GLsizei)w, (GLsizei)h);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
if (w <= h) {
glOrtho(-4.0, 4.0, -4.0 * (GLfloat)h / (GLfloat)w, 4.0 * (GLfloat)h / (GLfloat)w, -4.0, 4.0);
} else {
glOrtho(-4.0 * (GLfloat)w / (GLfloat)h, 4.0 * (GLfloat)w / (GLfloat)h, -4.0, 4.0, -4.0, 4.0);
}
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
}
int main(int argc, char *argv[])
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB | GLUT_DEPTH);
glutInitWindowSize(500, 500);
glutCreateWindow("Bezier Surface");
init();
glutDisplayFunc(display);
glutReshapeFunc(reshape);
glutMainLoop();
return 0;
}
```
该程序中通过定义`ctrlPoints`数组来表示Bezier曲面的控制点,使用OpenGL提供的函数进行绘制,其中`glMapGrid2f()`函数用于生成网格,`glEvalMesh2()`函数用于对网格进行绘制。可以根据需要修改控制点坐标来绘制不同形状的曲面。
阅读全文
相关推荐
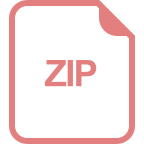
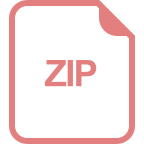










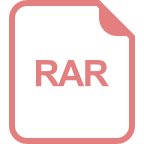
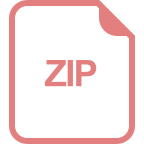
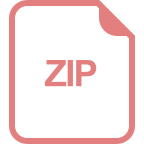
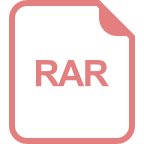
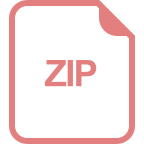
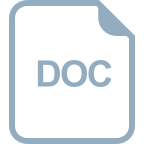