使用C++设计一个符合C语言语法的抽象语法树的结构,给出能构建整颗树的建树代码
时间: 2024-02-18 11:00:23 浏览: 136
可以使用C++中的类来表示抽象语法树节点,每个节点包含该节点的类型、值、子节点等信息。例如,可以定义一个基类 `ASTNode`,包含所有节点共有的属性和方法,然后再针对不同的节点类型定义不同的子类。
以下是一个简单的示例代码:
```cpp
#include <vector>
#include <string>
// 抽象语法树节点类型
enum class ASTNodeType {
Program,
Statement,
Expression,
NumberLiteral,
StringLiteral,
Identifier,
BinaryExpression
};
// 抽象语法树节点基类
class ASTNode {
public:
virtual ~ASTNode() = default;
ASTNodeType getType() const { return type_; }
virtual std::vector<ASTNode*> getChildren() const { return {}; }
protected:
ASTNode(ASTNodeType type) : type_(type) {}
private:
ASTNodeType type_;
};
// 数字字面量节点
class NumberLiteralNode : public ASTNode {
public:
NumberLiteralNode(double value) : ASTNode(ASTNodeType::NumberLiteral), value_(value) {}
double getValue() const { return value_; }
private:
double value_;
};
// 字符串字面量节点
class StringLiteralNode : public ASTNode {
public:
StringLiteralNode(const std::string& value) : ASTNode(ASTNodeType::StringLiteral), value_(value) {}
const std::string& getValue() const { return value_; }
private:
std::string value_;
};
// 标识符节点
class IdentifierNode : public ASTNode {
public:
IdentifierNode(const std::string& name) : ASTNode(ASTNodeType::Identifier), name_(name) {}
const std::string& getName() const { return name_; }
private:
std::string name_;
};
// 二元表达式节点
class BinaryExpressionNode : public ASTNode {
public:
BinaryExpressionNode(const std::string& op, ASTNode* left, ASTNode* right)
: ASTNode(ASTNodeType::BinaryExpression), op_(op), left_(left), right_(right) {}
const std::string& getOperator() const { return op_; }
ASTNode* getLeft() const { return left_; }
ASTNode* getRight() const { return right_; }
std::vector<ASTNode*> getChildren() const override { return {left_, right_}; }
private:
std::string op_;
ASTNode* left_;
ASTNode* right_;
};
// 构建抽象语法树
ASTNode* buildAST() {
// 例如,将代码 "1 + 2 * 3" 构建成以下抽象语法树
// +
// / \
// 1 *
// / \
// 2 3
auto* num1 = new NumberLiteralNode(1);
auto* num2 = new NumberLiteralNode(2);
auto* num3 = new NumberLiteralNode(3);
auto* mul = new BinaryExpressionNode("*", num2, num3);
auto* add = new BinaryExpressionNode("+", num1, mul);
return add;
}
```
调用 `buildAST()` 函数即可构建出一颗抽象语法树。当然,这只是一个简单的示例,实际中可能需要更多的节点类型和属性。
阅读全文
相关推荐
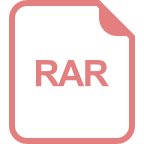
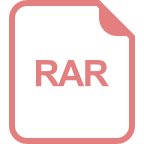
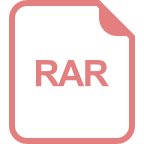
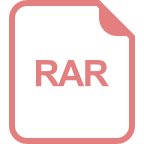
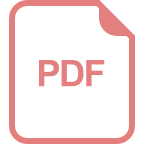
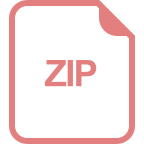
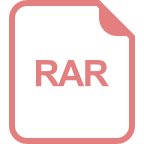
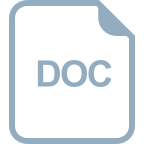
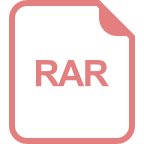
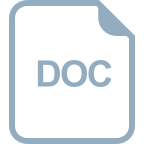
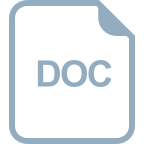