解读void student_information_load(struct information *stu) { //读入序号 fscanf(fp,"%d",&stu->number); //读入学号 fscanf(fp,"%s",stu->id); //读入姓名 fscanf(fp,"%s",stu->name); //读入性别 fscanf(fp,"%s",stu->gender); //读入班级 fscanf(fp,"%s",stu->clas); } void student_information_print(struct information *stu) { fputs(stu->name,fpp); fprintf(fpp,"\t"); fputs(stu->gender,fpp); fprintf(fpp,"\t"); fputs(stu->clas,fpp); fprintf(fpp,"\n"); }
时间: 2023-12-06 10:40:49 浏览: 30
这是两个函数,分别是读入学生信息和打印学生信息的函数。这里用到了结构体指针,结构体包含了学生的序号、学号、姓名、性别和班级等信息。
函数 `student_information_load()` 用于从文件中读取学生信息,输入参数为一个指向结构体的指针 `stu`。首先通过 `fscanf()` 函数读取学生的序号、学号、姓名、性别和班级等信息,然后将这些信息存储在结构体 `stu` 中。
函数 `student_information_print()` 用于将学生信息打印到文件中,输入参数也是一个指向结构体的指针 `stu`。函数中使用了 `fputs()` 和 `fprintf()` 函数将学生的姓名、性别和班级等信息写入到文件中。
这两个函数一般是作为学生信息管理系统的一部分,用于读取和打印学生信息。
相关问题
优化这段代码void student_information(struct stu **point) { //显示全部考生信息 FILE *fp; int i; struct stu *new; static struct stu *tail; if ((fp = fopen("C:/Users/MonnyX/Desktop/c/file2.dat", "r")) == NULL) { printf("打开文件失败!\n"); exit(0); } for (i = 1;i<=2; i++) { new = (struct stu *)malloc(sizeof(struct stu)); if (new == NULL) { printf("内存分配失败!\n"); exit(1); } fscanf(fp, "%d", &new->num); fscanf(fp, "%s", new->name); fscanf(fp, "%d", &new->age); fscanf(fp, "%s", new->sex); if (*point == NULL) { *point = new; new->next = NULL; } else { tail->next = new; new->next = NULL; } } tail = new; for(i=1;i<=2;i++){ printf("%d", new->num); printf("%s", new->name); printf("%d", new->age); printf("%s", new->sex); new=new->next; } fclose(fp); system("pause"); system("clear"); }
这段代码可以进行如下优化:
1. 将文件路径定义为常量或者宏,以便于修改和维护。
2. 将读取文件的代码封装成一个函数,可以复用。
3. 使用fgets()函数代替fscanf()函数,可以避免因为格式化字符串不匹配而导致的错误。
4. 将打印信息的代码封装成一个函数,可以复用。
5. 去掉不必要的变量和操作,简化代码结构。
6. 最好不要使用system()函数,因为它不可移植,可以使用跨平台的清屏函数。
下面是优化后的代码:
```
#define FILE_PATH "C:/Users/MonnyX/Desktop/c/file2.dat"
void read_student_information(struct stu **point) {
FILE *fp;
int i;
struct stu *new;
static struct stu *tail;
if ((fp = fopen(FILE_PATH, "r")) == NULL) {
printf("打开文件失败!\n");
exit(0);
}
for (i = 1; i <= 2; i++) {
new = (struct stu *)malloc(sizeof(struct stu));
if (new == NULL) {
printf("内存分配失败!\n");
exit(1);
}
char buf[1024];
fgets(buf, sizeof(buf), fp);
sscanf(buf, "%d %s %d %s", &new->num, new->name, &new->age, new->sex);
if (*point == NULL) {
*point = new;
new->next = NULL;
} else {
tail->next = new;
new->next = NULL;
}
tail = new;
}
fclose(fp);
}
void print_student_information(struct stu *point) {
while (point != NULL) {
printf("%d %s %d %s\n", point->num, point->name, point->age, point->sex);
point = point->next;
}
}
void student_information(struct stu **point) {
read_student_information(point);
print_student_information(*point);
clear_screen();
}
```
int i; typedef struct student { int num; char name[20]; int score[3]; float avg; }student; void Inputdata(student* stu) { printf("请输入新学生的信息:\n"); printf("num name s1 s2 s3\n"); scanf("%d %s %d %d %d", &(stu->num), stu->name, &(stu->score[0]), &(stu->score[1]), &(stu->score[2])); stu->avg = (stu->score[0] + stu->score[1] + stu->score[2]) / 3.0; } void readdate(student stu[]) { FILE* fp = fopen("stu_ sort.txt", "r"); if (fp == NULL) { printf("read stu_ sort file error!"); return -1; } for (i = 0; i < 5; i++)//从文件中读入数据 { fscanf(fp, "%d %s %d %d %d %f", &stu[i].num, stu[i].name, &stu[i].score[0], &stu[i].score[1], &stu[i].score[2], &stu[i].avg); } fclose(fp); } void Insertdata(student* oldstu, student* newstu,int n) { int pos = 0; while (pos < n) { if ((newstu->avg) < (oldstu[pos].avg)) break; pos++; } for (i = n; i > pos; i--) { memcpy(&oldstu[i],&oldstu[i-1], sizeof(student)); } memcpy(&oldstu[pos], newstu, sizeof(student)); } void writedate(student* stu) { FILE* fp = fopen("stu_new_sort.txt", "w"); if (fp == NULL) { printf("write file error!"); return -1; } for (i = 0; i < 6; i++)//使用fprint函数将结构体数组stu中的数据写入fp { fprintf(fp, "%d %s %d %d %d %f\n", stu[i].num, stu[i].name, stu[i].score[0], stu[i].score[1], stu[i].score[2], stu[i].avg); } fclose(fp); } int main() { student newstu;//首先定义一个新学生信息 Inputdata(&newstu);//输入这个新学生的信息 student oldstu[6]; readdate(oldstu);//调用函数从上一题排好序的文件中读入5个学生数据 Insertdata(oldstu, &newstu,5);//把新数据有序地插入到老数组中 writedate(oldstu);//把含有新数据的数组写入到新文件 return 0; }改写成正确的
int i;
typedef struct student {
int num;
char name[20];
int score[3];
float avg;
} student;
void Inputdata(student* stu) {
printf("请输入新学生的信息:\n");
printf("num name s1 s2 s3\n");
scanf("%d %s %d %d %d", &(stu->num), stu->name, &(stu->score[0]), &(stu->score[1]), &(stu->score[2]));
stu->avg = (stu->score[0] + stu->score[1] + stu->score[2]) / 3.0;
}
int readdate(student stu[]) {
FILE* fp = fopen("stu_sort.txt", "r");
if (fp == NULL) {
printf("read stu_sort file error!");
return -1;
}
for (i = 0; i < 5; i++) {
fscanf(fp, "%d %s %d %d %d %f", &stu[i].num, stu[i].name, &stu[i].score[0], &stu[i].score[1], &stu[i].score[2], &stu[i].avg);
}
fclose(fp);
return 0;
}
void Insertdata(student* oldstu, student* newstu, int n) {
int pos = 0;
while (pos < n) {
if ((newstu->avg) < (oldstu[pos].avg))
break;
pos++;
}
for (i = n; i > pos; i--) {
memcpy(&oldstu[i], &oldstu[i - 1], sizeof(student));
}
memcpy(&oldstu[pos], newstu, sizeof(student));
}
int writedate(student* stu) {
FILE* fp = fopen("stu_new_sort.txt", "w");
if (fp == NULL) {
printf("write file error!");
return -1;
}
for (i = 0; i < 6; i++) {
fprintf(fp, "%d %s %d %d %d %f\n", stu[i].num, stu[i].name, stu[i].score[0], stu[i].score[1], stu[i].score[2], stu[i].avg);
}
fclose(fp);
return 0;
}
int main() {
student newstu;
Inputdata(&newstu);
student oldstu[6];
if (readdate(oldstu) == -1) {
return -1;
}
Insertdata(oldstu, &newstu, 5);
if (writedate(oldstu) == -1) {
return -1;
}
return 0;
}
相关推荐
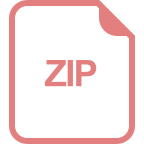
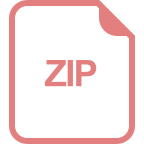













