python实现de_casteljau算法构造bezier曲线
时间: 2023-09-30 14:12:57 浏览: 150
de Casteljau算法是用于构造Bezier曲线的一种方法,其基本思想是将控制点逐步插值,最终得到Bezier曲线上的点。下面是Python实现de Casteljau算法构造Bezier曲线的代码:
```python
import numpy as np
import matplotlib.pyplot as plt
def de_casteljau(points, t):
"""
Compute a point on the Bezier curve using the de Casteljau algorithm.
:param points: list of control points
:param t: parameter value between 0 and 1
:return: point on the Bezier curve
"""
n = len(points) - 1
b = np.copy(points)
for r in range(1, n+1):
for i in range(n-r+1):
b[i] = (1-t)*b[i] + t*b[i+1]
return b[0]
def bezier_curve(points, num=100):
"""
Compute the Bezier curve given a list of control points.
:param points: list of control points
:param num: number of points to sample on the curve
:return: list of points on the Bezier curve
"""
curve = []
for i in range(num):
t = i / (num-1)
curve.append(de_casteljau(points, t))
return curve
# Example usage
points = np.array([[0, 0], [1, 3], [2, -1], [3, 2]])
curve = bezier_curve(points)
# Plot the control points and the curve
plt.plot(points[:,0], points[:,1], 'bo-', label='Control Points')
plt.plot([p[0] for p in curve], [p[1] for p in curve], 'r-', label='Bezier Curve')
plt.legend()
plt.show()
```
在这个示例中,我们定义了四个控制点,并使用`bezier_curve`函数计算曲线上的100个点。然后,我们将控制点和曲线都绘制到图表中。运行代码可以看到Beizer曲线的图像。
阅读全文
相关推荐
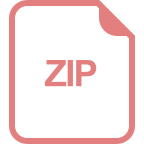

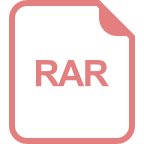
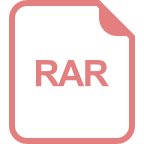
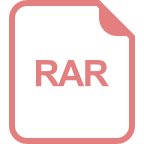
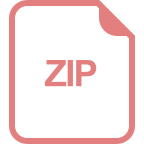
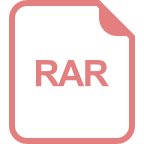
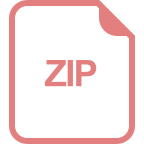
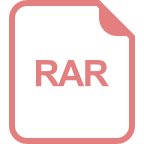
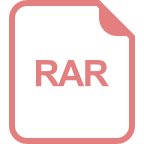
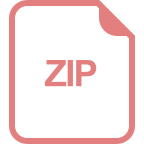
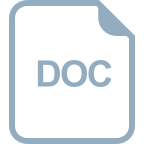
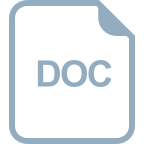
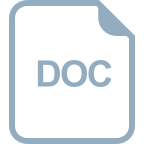
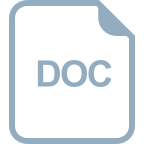


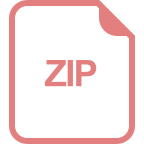