定义一个表示点的类Point,类中有两个私有成员变量x和y;使用成员函数和友元函数两种方法,为该类重载以下运算符:加+ 增量++(包括前增和后增) == 主函数中分别使用以上运算符。
时间: 2023-06-27 17:07:27 浏览: 78
使用成员函数重载运算符:
```cpp
#include<iostream>
using namespace std;
class Point{
private:
int x, y;
public:
Point(int x=0, int y=0):x(x), y(y){}
Point operator+(const Point& p){
return Point(x+p.x, y+p.y);
}
Point& operator++(){ //前增量
x++;
y++;
return *this;
}
Point operator++(int){ //后增量
Point old = *this;
x++;
y++;
return old;
}
bool operator==(const Point& p){
return x==p.x && y==p.y;
}
friend ostream& operator<<(ostream& os, const Point& p){
os<<"("<<p.x<<", "<<p.y<<")";
return os;
}
};
int main(){
Point p1(1, 2), p2(3, 4), p3;
p3 = p1 + p2;
cout<<p1<<" + "<<p2<<" = "<<p3<<endl;
if (p1 == p2){
cout<<p1<<" == "<<p2<<endl;
}else{
cout<<p1<<" != "<<p2<<endl;
}
++p1;
cout<<"++"<<p1<<" = "<<p1<<endl;
p2++;
cout<<p2<<"++ = "<<p2<<endl;
return 0;
}
```
使用友元函数重载运算符:
```cpp
#include<iostream>
using namespace std;
class Point{
private:
int x, y;
public:
Point(int x=0, int y=0):x(x), y(y){}
friend Point operator+(const Point& p1, const Point& p2){
return Point(p1.x+p2.x, p1.y+p2.y);
}
Point& operator++(){ //前增量
x++;
y++;
return *this;
}
Point operator++(int){ //后增量
Point old = *this;
x++;
y++;
return old;
}
friend bool operator==(const Point& p1, const Point& p2){
return p1.x==p2.x && p1.y==p2.y;
}
friend ostream& operator<<(ostream& os, const Point& p){
os<<"("<<p.x<<", "<<p.y<<")";
return os;
}
};
int main(){
Point p1(1, 2), p2(3, 4), p3;
p3 = p1 + p2;
cout<<p1<<" + "<<p2<<" = "<<p3<<endl;
if (p1 == p2){
cout<<p1<<" == "<<p2<<endl;
}else{
cout<<p1<<" != "<<p2<<endl;
}
++p1;
cout<<"++"<<p1<<" = "<<p1<<endl;
p2++;
cout<<p2<<"++ = "<<p2<<endl;
return 0;
}
```
相关推荐
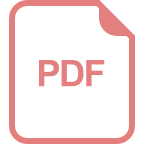
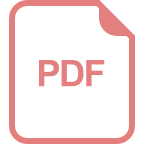
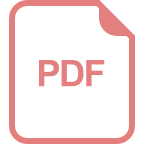














