设计一个名为rectangle的矩形类,其私有属性为矩形的左上角和右下角两个点的坐标,成员函数能计算和输出矩形的周长,并统计程序中目前存在矩形类对象的个数,然后写一个友元函数求矩形的面积。
时间: 2023-05-31 14:18:19 浏览: 150
### 回答1:
好的,下面是中文回复:
首先,我们可以定义一个名为Rectangle的类,其中包含私有属性left_top和right_bottom,分别表示矩形的左上角和右下角的坐标。我们可以在类中定义一个构造函数,用于初始化这两个属性。此外,我们还需要定义一个静态成员变量count,用于统计程序中目前存在矩形类对象的个数。
接下来,我们需要实现一个成员函数getPerimeter,用于计算和输出矩形的周长。这个函数可以通过计算矩形的长和宽,然后将它们相加得到周长。
最后,我们需要实现一个友元函数getArea,用于计算矩形的面积。由于这个函数需要访问矩形的私有属性,所以我们需要将它声明为Rectangle类的友元函数。
下面是完整的代码示例:
```cpp
#include <iostream>
using namespace std;
class Rectangle {
private:
struct Point {
int x;
int y;
} left_top, right_bottom;
static int count;
public:
Rectangle(int x1, int y1, int x2, int y2) {
left_top.x = x1;
left_top.y = y1;
right_bottom.x = x2;
right_bottom.y = y2;
count++;
}
void getPerimeter() {
int length = right_bottom.x - left_top.x;
int width = right_bottom.y - left_top.y;
int perimeter = 2 * (length + width);
cout << "矩形的周长为:" << perimeter << endl;
}
friend int getArea(Rectangle r);
static int getCount() {
return count;
}
};
int Rectangle::count = ;
int getArea(Rectangle r) {
int length = r.right_bottom.x - r.left_top.x;
int width = r.right_bottom.y - r.left_top.y;
int area = length * width;
return area;
}
int main() {
Rectangle r1(, , 5, 5);
Rectangle r2(, , 10, 10);
r1.getPerimeter();
r2.getPerimeter();
cout << "目前存在 " << Rectangle::getCount() << " 个矩形类对象" << endl;
cout << "矩形的面积为:" << getArea(r1) << endl;
return ;
}
```
在上面的代码中,我们定义了一个名为Point的结构体,用于表示矩形的左上角和右下角的坐标。然后,我们在Rectangle类中定义了一个静态成员变量count,用于统计程序中目前存在矩形类对象的个数。接着,我们实现了一个构造函数,用于初始化矩形的左上角和右下角的坐标,并在构造函数中将count加1。然后,我们实现了一个成员函数getPerimeter,用于计算和输出矩形的周长。最后,我们实现了一个友元函数getArea,用于计算矩形的面积。在main函数中,我们创建了两个矩形类对象r1和r2,并分别调用它们的getPerimeter函数。然后,我们输出了目前存在的矩形类对象的个数,并调用了getArea函数计算矩形r1的面积。
### 回答2:
设计一个名为rectangle的矩形类,可以使用C++语言中的面向对象编程的思想来实现。
首先,矩形的私有属性为左上角和右下角两个点的坐标,可以用两个结构体来表示,如下所示:
struct Point {
double x;
double y;
};
class Rectangle {
private:
Point topLeft; // 左上角点坐标
Point bottomRight; // 右下角点坐标
static int count; // 类对象的个数
public:
Rectangle(Point tl, Point br); // 构造函数
double perimeter(); // 计算周长
static int getCount(); // 获取对象个数
friend double area(Rectangle rec); // 友元函数,计算面积
};
其中,静态变量count用于统计程序中目前存在矩形类对象的个数。
接下来,实现构造函数和计算周长的成员函数:
Rectangle::Rectangle(Point tl, Point br)
{
topLeft = tl;
bottomRight = br;
count++;
}
double Rectangle::perimeter()
{
double width = bottomRight.x - topLeft.x;
double height = topLeft.y - bottomRight.y;
return (width + height) * 2;
}
其中,计算周长的公式为周长=2*(矩形宽+矩形高)。
接着,实现获取对象个数的静态函数:
int Rectangle::getCount()
{
return count;
}
最后,定义友元函数计算矩形的面积:
double area(Rectangle rec)
{
double width = rec.bottomRight.x - rec.topLeft.x;
double height = rec.topLeft.y - rec.bottomRight.y;
return width * height;
}
至此,矩形类的设计完毕。
完整代码如下所示:
#include <iostream>
using namespace std;
struct Point {
double x;
double y;
};
class Rectangle {
private:
Point topLeft; // 左上角点坐标
Point bottomRight; // 右下角点坐标
static int count; // 类对象的个数
public:
Rectangle(Point tl, Point br); // 构造函数
double perimeter(); // 计算周长
static int getCount(); // 获取对象个数
friend double area(Rectangle rec); // 友元函数,计算面积
};
Rectangle::Rectangle(Point tl, Point br)
{
topLeft = tl;
bottomRight = br;
count++;
}
double Rectangle::perimeter()
{
double width = bottomRight.x - topLeft.x;
double height = topLeft.y - bottomRight.y;
return (width + height) * 2;
}
int Rectangle::count = 0;
int Rectangle::getCount()
{
return count;
}
double area(Rectangle rec)
{
double width = rec.bottomRight.x - rec.topLeft.x;
double height = rec.topLeft.y - rec.bottomRight.y;
return width * height;
}
int main()
{
Point tl = {0, 5};
Point br = {10, 0};
Rectangle r(tl, br);
cout << "周长:" << r.perimeter() << endl;
cout << "对象个数:" << Rectangle::getCount() << endl;
cout << "面积:" << area(r) << endl;
return 0;
}
### 回答3:
题目要求我们设计一个名为 rectangle 的矩形类,并给出它的私有属性和成员函数。既然是矩形类,那么我们需要知道矩形的几何信息,包括矩形的左上角和右下角两个点的坐标。因此,我们可以用两个 Point 类型的变量来表示矩形的两个点:
class Point {
public:
Point(int x = 0, int y = 0) : m_x(x), m_y(y) {}
int x() const { return m_x; }
int y() const { return m_y; }
private:
int m_x;
int m_y;
};
class Rectangle {
public:
Rectangle(const Point& left_top, const Point& right_bottom)
: m_leftTop(left_top), m_rightBottom(right_bottom) {
++s_count; // 统计矩形对象的个数
}
~Rectangle() {
--s_count;
}
double perimeter() const {
int width = abs(m_rightBottom.x() - m_leftTop.x());
int height = abs(m_rightBottom.y() - m_leftTop.y());
return 2 * (width + height);
}
friend double area(const Rectangle& rect);
private:
Point m_leftTop;
Point m_rightBottom;
static int s_count; // 统计矩形对象的个数
};
int Rectangle::s_count = 0;
在矩形类的定义中,我们使用了一个静态成员变量 s_count 来记录当前程序中创建了多少个矩形对象,并且在构造函数中对其进行自增操作,在析构函数中对其进行自减操作。
成员函数 perimeter() 的作用是计算和输出矩形的周长。我们知道,矩形的周长等于 2×(宽+高),可以通过公式算出来。其中宽和高可以通过左上角和右下角的坐标差值来计算。
注意,我们将 area() 函数声明为了矩形类的友元函数,这是因为 area() 函数需要访问矩形类的私有属性。
接下来,我们来实现 area() 函数:
double area(const Rectangle& rect) {
int width = abs(rect.m_rightBottom.x() - rect.m_leftTop.x());
int height = abs(rect.m_rightBottom.y() - rect.m_leftTop.y());
return width * height;
}
area() 函数的作用是计算矩形的面积,根据矩形的定义,面积等于宽×高,同样可以通过矩形的两个点坐标来计算。
以上就是这道题的解答,我们完成了一个简单的矩形类,并设定了成员函数和友元函数来计算矩形的周长和面积,同时记录了程序中创建的矩形对象的个数。
阅读全文
相关推荐
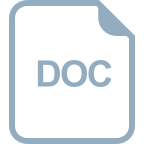
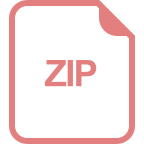
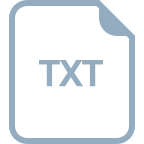















