python 函数中使用字典
时间: 2024-01-17 17:06:11 浏览: 46
在 Python 函数中使用字典可以方便地处理参数、返回值等数据。以下是一些使用字典的例子:
1. 使用字典作为函数参数
```python
def print_info(name, age, **kwargs):
print(f"Name: {name}")
print(f"Age: {age}")
print("Other Info:")
for key, value in kwargs.items():
print(f"{key}: {value}")
# 调用函数,传入字典作为参数
info_dict = {"gender": "male", "hometown": "Beijing"}
print_info("John", 25, **info_dict)
```
输出结果:
```
Name: John
Age: 25
Other Info:
gender: male
hometown: Beijing
```
2. 使用字典作为函数返回值
```python
def get_person_info():
info_dict = {"name": "John", "age": 25, "gender": "male"}
return info_dict
# 调用函数,获取字典作为返回值
person_info = get_person_info()
print(person_info["name"]) # 输出 John
print(person_info["age"]) # 输出 25
print(person_info["gender"]) # 输出 male
```
输出结果:
```
John
25
male
```
3. 使用字典存储函数的状态
```python
def counter():
count_dict = {"count": 0}
def add():
count_dict["count"] += 1
return count_dict["count"]
def reset():
count_dict["count"] = 0
return {"add": add, "reset": reset}
# 创建一个计数器
c = counter()
print(c["add"]()) # 输出 1
print(c["add"]()) # 输出 2
c["reset"]()
print(c["add"]()) # 输出 1
```
输出结果:
```
1
2
1
```
在上面的例子中,`counter()` 函数返回一个字典,包含了两个函数 `add()` 和 `reset()`,以及一个字典 `count_dict`。每次调用 `add()` 函数,都会使计数器加一,并返回当前计数值。调用 `reset()` 函数可以重置计数器。
相关推荐
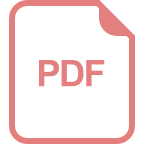














