qt override
时间: 2023-07-05 14:22:06 浏览: 66
`override`是C++11中的一个关键字,用于在子类中重写(override)基类中的虚函数,并显式地表明该函数是重写基类中的虚函数。
在Qt中,`override`关键字通常用于重写Qt类中的虚函数。当你重写一个Qt类中的虚函数时,建议使用`override`关键字,因为这可以有效地避免由于函数签名不一致等问题导致的编译错误。
例如,在重写`QGraphicsView`的`mousePressEvent`函数时,建议使用`override`关键字:
```cpp
class MyGraphicsView : public QGraphicsView
{
protected:
void mousePressEvent(QMouseEvent *event) override
{
// ...
}
};
```
在上面的代码中,我们使用了`override`关键字来明确表示`mousePressEvent`函数是在子类中重写`QGraphicsView`中的虚函数。
需要注意的是,在使用`override`关键字时,必须保证子类中的函数签名与基类中的虚函数的签名完全一致,否则会导致编译错误。
相关问题
qt5 override
在Qt 5中,`override`关键字用于标记函数是覆盖其基类中的虚函数。它是C++11中的一个关键字,用于明确地表示一个函数是覆盖其基类中的虚函数,这有助于提高代码的可读性和可维护性。
使用`override`关键字的好处在于,如果您尝试覆盖不存在于基类中的虚函数,编译器将会抛出一个错误,从而避免一些常见的错误。
以下是一个简单的示例,演示如何在Qt 5中使用`override`关键字:
```cpp
class Base {
public:
virtual void foo() {}
};
class Derived : public Base {
public:
void foo() override {}
};
```
在这个例子中,`Derived`类覆盖了`Base`类中的`foo`函数,并使用了`override`关键字来明确地表示这一点。
qt中 override作用
在Qt中,`override`是C++11中的一个关键字,用于显式地指示一个虚函数是重写基类中的虚函数。它的作用是帮助程序员更好地理解代码,并且在编译时进行类型检查,避免出现不必要的错误。
使用`override`关键字,可以让编译器检查子类中的重写函数是否与基类中的虚函数完全匹配,包括函数名称、参数列表和返回类型。如果不完全匹配,则编译器会发出错误提示,让程序员进行修复。
示例代码:
```cpp
class A {
public:
virtual void foo() {}
};
class B : public A {
public:
void foo() override {}
};
```
在上面的代码中,`B`类重写了`A`类中的`foo`函数,并使用`override`关键字显式地指示了这一点。编译器会检查`B::foo`函数是否与`A::foo`函数完全匹配,如果不匹配则会发出错误提示。
总之,`override`关键字可以提高程序的可读性和健壮性,建议在Qt中的虚函数重写中使用它。
相关推荐
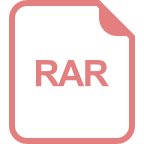
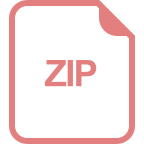












