编写一个程序,在屏幕中出现一个圆心沿正弦曲线轨迹移动的小圆,要求每隔四分之一周期,圆的填充色和圆的周边颜色都发生变化(颜色自己选取),同时,圆的半径在四分之一周期之内由正弦曲线幅值的0.2倍至0.6倍线性增长。小圆能从左向右再从右向左不间断移动。用c语言的windows库
时间: 2023-05-13 21:06:32 浏览: 143
以下是一个可能的实现:
```c
#include <windows.h>
#include <math.h>
#define PI 3.14159265358979323846
LRESULT CALLBACK WndProc(HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow)
{
WNDCLASS wc = {0};
wc.lpfnWndProc = WndProc;
wc.hInstance = hInstance;
wc.hCursor = LoadCursor(NULL, IDC_ARROW);
wc.hbrBackground = (HBRUSH)(COLOR_WINDOW + 1);
wc.lpszClassName = TEXT("MyWindowClass");
RegisterClass(&wc);
HWND hWnd = CreateWindow(TEXT("MyWindowClass"), TEXT("Moving Circle"), WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT, CW_USEDEFAULT, 640, 480, NULL, NULL, hInstance, NULL);
ShowWindow(hWnd, nCmdShow);
UpdateWindow(hWnd);
MSG msg;
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return (int)msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam)
{
static int cxClient, cyClient;
static int cxCenter, cyCenter;
static int cxRadius, cyRadius;
static int cxOffset, cyOffset;
static COLORREF fillColor, borderColor;
static double t = 0.0;
switch (message)
{
case WM_CREATE:
fillColor = RGB(255, 0, 0);
borderColor = RGB(0, 255, 0);
cxRadius = cyRadius = (int)(0.2 * cyClient);
cxOffset = cyOffset = 0;
SetTimer(hWnd, 1, 20, NULL);
break;
case WM_SIZE:
cxClient = LOWORD(lParam);
cyClient = HIWORD(lParam);
cxCenter = cxClient / 2;
cyCenter = cyClient / 2;
break;
case WM_TIMER:
t += 0.05;
if (t > 2 * PI)
t -= 2 * PI;
cxRadius = (int)(0.2 * cyClient + 0.4 * cyClient * (1 + sin(t)));
if (cxOffset < cxClient)
cxOffset += 2;
else
cxOffset = -cxRadius;
InvalidateRect(hWnd, NULL, TRUE);
break;
case WM_PAINT:
{
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hWnd, &ps);
HBRUSH hFillBrush = CreateSolidBrush(fillColor);
HBRUSH hOldBrush = (HBRUSH)SelectObject(hdc, hFillBrush);
HPEN hBorderPen = CreatePen(PS_SOLID, 2, borderColor);
HPEN hOldPen = (HPEN)SelectObject(hdc, hBorderPen);
Ellipse(hdc, cxCenter - cxRadius + cxOffset, cyCenter - cyRadius + cyOffset,
cxCenter + cxRadius + cxOffset, cyCenter + cyRadius + cyOffset);
SelectObject(hdc, hOldBrush);
DeleteObject(hFillBrush);
SelectObject(hdc, hOldPen);
DeleteObject(hBorderPen);
EndPaint(hWnd, &ps);
}
break;
case WM_DESTROY:
KillTimer(hWnd, 1);
PostQuitMessage(0);
break;
default:
return DefWindowProc(hWnd, message, wParam, lParam);
}
return 0;
}
```
这个程序使用了 Windows 库,创建了一个窗口,并在窗口中绘制了一个圆,圆心沿正弦曲线轨迹移动,同时圆的填充色和周边颜色每隔四分之一周期发生变化,圆的半径在四分之一周期内由正弦曲线幅值的0.2倍至0.6倍线性增长。程序使用了定时器来控制圆的移动和变化。
阅读全文
相关推荐
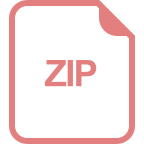
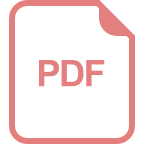
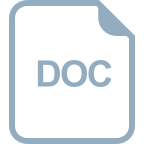















