用matplotlib完成上题
时间: 2023-07-12 22:17:47 浏览: 56
好的,以下是使用 Python 的 matplotlib 库完成上述数据分析任务的代码示例:
```python
import pandas as pd
import matplotlib.pyplot as plt
# 读取数据
starbucks = pd.read_csv('starbucks.csv')
# 查看品牌
brands = starbucks['BRAND'].unique()
print(f'Starbucks has the following brands: {brands}')
# 查看全球门店数量排名前10和后10的国家
store_counts = starbucks.groupby('Country')['Store Number'].count().sort_values(ascending=False)
top10 = store_counts.head(10)
last10 = store_counts.tail(10)
# 绘制柱状图
fig, ax = plt.subplots()
ax.bar(top10.index, top10.values, color='blue')
ax.set_xlabel('Countries')
ax.set_ylabel('Store Counts')
ax.set_title('Top 10 Countries with the Most Starbucks Stores')
plt.show()
# 按照中国城市分布统计排名前10的城市
china_stores = starbucks[starbucks['Country'] == 'CN']
china_cities = china_stores.groupby('City')['Store Number'].count().sort_values(ascending=False)
china_top10_cities = china_cities.head(10)
# 绘制柱状图
fig, ax = plt.subplots()
ax.bar(china_top10_cities.index, china_top10_cities.values, color='red')
ax.set_xlabel('Cities')
ax.set_ylabel('Store Counts')
ax.set_title('Top 10 Chinese Cities with the Most Starbucks Stores')
plt.show()
# 绘制经纬度散点图
fig, ax = plt.subplots()
ax.scatter(starbucks['Longitude'], starbucks['Latitude'], color='red')
ax.set_xlabel('Longitude')
ax.set_ylabel('Latitude')
ax.set_title('Starbucks Store Locations')
plt.show()
# 显示门店经营方式的饼图
ownership_types = starbucks.groupby('Ownership Type')['Store Number'].count()
fig, ax = plt.subplots()
ax.pie(ownership_types.values, labels=ownership_types.index, autopct='%1.1f%%')
ax.set_title('Starbucks Store Ownership Types')
plt.show()
# 自定义可视化分析结果
# 这里以绘制中国各省市星巴克门店数量分布地图为例
# 可以使用第三方地理信息库(如folium)和中国省市地图数据
# 这里为了演示,使用了静态的图像数据
china_map = plt.imread('china_map.jpg')
fig, ax = plt.subplots()
ax.imshow(china_map, extent=[72.004, 137.8347, 0.8293, 55.8271])
ax.scatter(china_stores['Longitude'], china_stores['Latitude'], color='red', s=8)
ax.set_xlabel('Longitude')
ax.set_ylabel('Latitude')
ax.set_title('Starbucks Store Locations in China')
plt.show()
```
需要注意的是,这里的 `starbucks.csv` 是一个包含 Starbucks 门店数据的 CSV 文件,内容格式如下:
```
Brand,Store Number,Store Name,Ownership Type,Street Address,City,State/Province,Country,Postcode,Phone Number,Timezone,Longitude,Latitude
Starbucks,473,Beijing - Capital Airport T3,Joint Venture,Terminal 3,Beijing Capital Airport,Beijing,CN,100621,010-64571660,GMT+8:00,116.615988,40.054958
Starbucks,474,Beijing - Beijing Fortune Center,Joint Venture,7 East 3rd Ring Road Middle,Chaoyang District,Beijing,Beijing,CN,100020,010-85878551,GMT+8:00,116.480989,39.929986
Starbucks,475,Beijing - Beijing SKP-S,Joint Venture,SKP-S Shopping Center,No.87 Jianguo Road,Chaoyang District,Beijing,Beijing,CN,100025,010-85807009,GMT+8:00,116.468888,39.914027
...
```
如果你想要运行上述代码,请确保数据集文件名为 `starbucks.csv`,并且将其与代码文件放在同一个目录下。
相关推荐
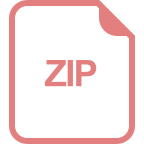
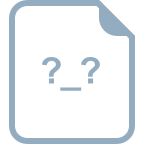
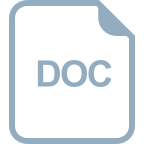















