C语言实现查找二叉树中某个位置结点的值
时间: 2024-10-28 20:12:47 浏览: 8
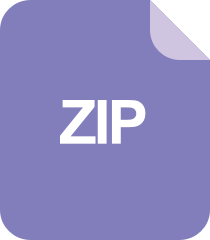
用C语言实现的二叉树
在C语言中,查找二叉搜索树中某个位置的节点通常涉及递归函数。假设我们有一个`struct TreeNode`表示二叉树节点,包含`int val`存储节点值,`struct TreeNode *left`和`struct TreeNode *right`分别指向左子树和右子树。以下是一个简单的查找函数实现:
```c
typedef struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
TreeNode* searchBST(TreeNode* root, int target) {
if (root == NULL || root->val == target) {
return root; // 找到或结束递归
}
if (target < root->val) {
// 目标比当前小,向左子树查找
return searchBST(root->left, target);
} else {
// 目标比当前大,向右子树查找
return searchBST(root->right, target);
}
}
```
在这个函数中,如果找到匹配的目标值,返回该节点指针;如果没有找到,返回NULL。
阅读全文
相关推荐
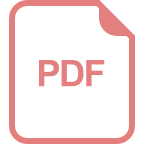
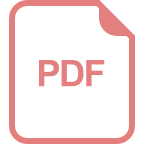















