你可以给我举个例子吗 用代码
时间: 2024-12-22 20:21:47 浏览: 5
当然可以,这里是一个简单的Python代码示例,展示了如何使用类(Class)实现多态:
```python
class Animal:
def make_sound(self):
pass
# 定义两个继承自Animal的子类
class Dog(Animal):
def make_sound(self):
return "Woof!"
class Cat(Animal):
def make_sound(self):
return "Meow!"
# 创建实例并调用make_sound方法
animals = [Dog(), Cat()]
for animal in animals:
print(animal.make_sound()) # 输出结果会是"Woof!" 和 "Meow!"
```
在这个例子中,`Animal`是父类,`Dog`和`Cat`是其子类。虽然它们都有一个共同的方法`make_sound()`,但是每个子类都实现了自己的版本。这就是多态的一个体现,我们通过相同的函数名调用,但实际的行为取决于对象的实际类型。
相关问题
我不理解,你能用代码举个例子吗?
好的,让我用代码来解释一下。
首先,我们来看看OpenAI公司开发的ChatGPT:
```python
import openai
# Set up the API key
openai.api_key = "YOUR_API_KEY"
# Define the prompt and generate the response
prompt = "Hi, how are you?"
response = openai.Completion.create(
engine="davinci",
prompt=prompt,
max_tokens=30
)
# Print the response
print(response.choices[0].text)
```
这是一个使用OpenAI的API进行对话的Python代码。它使用了OpenAI公司开发的GPT模型来生成回复。
现在,让我们来看看CSDN开发的ChitGPT:
```python
import torch
from transformers import GPT2Tokenizer, GPT2LMHeadModel
# Load the pre-trained model and tokenizer
tokenizer = GPT2Tokenizer.from_pretrained('chinese-electra-large-discriminator')
model = GPT2LMHeadModel.from_pretrained('chinese-electra-large-discriminator')
# Define the prompt and generate the response
prompt = "你好,你叫什么名字?"
input_ids = tokenizer.encode(prompt, return_tensors='pt')
output = model.generate(input_ids, max_length=50, do_sample=True)
response = tokenizer.decode(output[0], skip_special_tokens=True)
# Print the response
print(response)
```
这是一个使用CSDN开发的ChitGPT进行对话的Python代码。它使用了CSDN开发的GPT模型来生成回复。
可以看到,这两个代码段使用不同的GPT模型和API来进行对话,它们是独立开发的,没有直接关联。
你能用git cherry pick这行代码给我举些例子吗
可以的,git cherry-pick 是一个命令,用于将一个分支上的单个提交应用到另一个分支上。例如,如果我们想将分支 A 上的某个提交应用到分支 B 上,可以使用 git cherry-pick 命令。具体的命令格式为:git cherry-pick <commit-hash>。其中,<commit-hash> 是要应用的提交的哈希值。
阅读全文
相关推荐
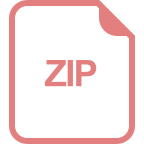
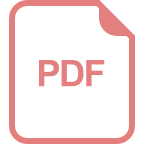
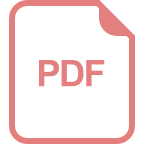













