ValueError: cannot reshape array of size 0 into shape (0,newaxis)
时间: 2024-05-03 20:18:56 浏览: 319
这个错误通常表示你正在尝试将一个大小为0的数组进行reshape操作,而这是不允许的。你需要检查一下你的代码,确保你正在操作的数组是非空的。
另外,你也可以在进行reshape操作之前,先打印一下数组的大小,以确保它不为空。你可以使用以下代码来打印数组的大小:
```
import numpy as np
arr = np.array([]) # 假设这是你的数组
print(arr.shape)
```
如果打印出来的是(0,),那么说明你的数组是空的,你需要在对它进行reshape操作之前先填充数据。
相关问题
ValueError: cannot reshape array of size 0 into shape (80,60)
This error occurs when you try to reshape an array into a shape that is incompatible with its current size. In this case, you are trying to reshape an array of size 0 into a shape of (80,60), which is not possible.
There are a few possible reasons why this error might occur:
1. Incorrect input: It's possible that the input data you are trying to reshape is empty or missing some values.
2. Incorrect dimensions: It's possible that the dimensions of your array are not what you expect them to be. For example, if you have a 1D array with 4800 elements, you could reshape it into a 2D array with shape (80, 60), but if you have a 1D array with only 10 elements, you cannot reshape it into a 2D array with shape (80, 60).
3. Incorrect reshape operation: It's possible that you are using the reshape() function incorrectly. Make sure that you are passing in the correct arguments and that the dimensions you are trying to reshape to are compatible with the size of the array.
To fix the error, you should check your input data and make sure it is not empty or missing any values. You should also double-check the dimensions of your array and make sure they are what you expect them to be. Finally, make sure that you are using the reshape() function correctly and passing in the correct arguments.
ValueError: cannot reshape array of size 3712000 into shape (500,1964)
该错误表明数据集 `E_h_cnn_7424.mat` 中的数组大小为 3712000,无法重塑为指定的形状 `(500, 1964)`。这是因为目标形状的元素总数(500 * 1964 = 982000)与原数组的元素总数不匹配。
### 解决方法:
1. **检查数据源**:确认 `E_h_cnn_7424.mat` 文件中的数据是否正确,并且其形状是否符合预期。
2. **调整目标形状**:如果数据是正确的,但确实需要重塑,可以尝试找到一个合适的形状,使得元素总数与原数组一致。例如,可以选择 `(1964, 1940)` 或其他适合的形状。
3. **数据预处理**:如果数据需要特定的形状,考虑在加载后进行适当的裁剪或填充操作,以使其符合所需的形状。
### 示例代码:
```python
import numpy as np
from scipy.io import loadmat
# 加载数据
mat = loadmat('E_h_cnn_7424.mat')
data = mat['E_h_cnn_7424']
# 检查数据形状
print(f"Original data shape: {data.shape}")
# 调整目标形状
target_shape = (500, 1964)
# 如果数据大小不符合目标形状,进行裁剪或填充
if data.size != target_shape[0] * target_shape[1]:
if data.size > target_shape[0] * target_shape[1]:
# 裁剪数据
data = data[:target_shape[0], :target_shape[1]]
else:
# 填充数据
padded_data = np.zeros(target_shape)
padded_data[:data.shape[0], :data.shape[1]] = data
data = padded_data
# 重新检查数据形状
print(f"Reshaped data shape: {data.shape}")
```
通过上述步骤,你可以解决数组大小不匹配的问题,并确保数据能够成功重塑为目标形状。
阅读全文
相关推荐
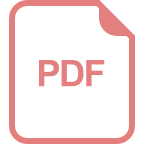
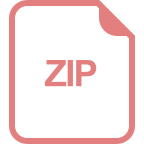
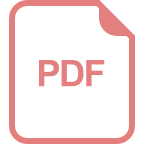













