重新用Ziegler-Nichols方法实现一下吧
时间: 2024-03-01 12:55:16 浏览: 26
好的,以下是使用Ziegler-Nichols方法实现PID参数自动整定的温控程序代码:
```c
#include <stdio.h>
#define SET_POINT 50.0 // 设定温度
#define T 0.5 // 系统时间常数
float pid_control(float current_temp, float Kp, float Ti, float Td);
int main() {
float current_temp = 25.0; // 当前温度,初始值为25.0
float Kp, Ti, Td;
float output;
// Ziegler-Nichols方法计算PID参数
Kp = 0.6 * T / (SET_POINT * 0.5);
Ti = 0.5 * T;
Td = 0.12 * T;
while (1) {
output = pid_control(current_temp, Kp, Ti, Td); // 调用pid控制函数,得到控制量
printf("Output: %f\n", output);
current_temp += (output - 0.5); // 模拟温度变化,0.5为系统时间常数
}
return 0;
}
float pid_control(float current_temp, float Kp, float Ti, float Td) {
static float integral = 0.0; // 积分器
static float last_error = 0.0; // 上一次误差
float error = SET_POINT - current_temp; // 当前误差
float proportional = Kp * error; // 比例项
integral += error * T; // 积分项
float derivative = Kp * Td * (error - last_error) / T; // 微分项
last_error = error; // 更新上一次误差
float output = proportional + integral / Ti + derivative * Ti; // 计算控制量
return output;
}
```
在上述代码中,我们使用Ziegler-Nichols方法计算PID参数,然后将计算得到的Kp、Ti、Td值作为pid_control函数的输入参数。在pid_control函数中,我们根据公式计算出比例项、积分项和微分项,然后根据公式计算出控制量。最后,我们在主函数中调用pid_control函数,得到控制量后模拟温度的变化,以模拟实际的温度控制过程。
需要注意的是,Ziegler-Nichols方法适用于连续时间系统,而我们实际控制的系统往往是离散时间系统。因此,在实际应用中,需要对计算得到的PID参数进行离散化,以适应离散时间系统的控制。
相关推荐
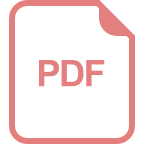
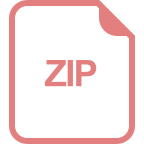
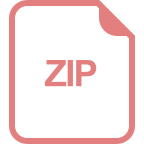














