生成一个运用遗传算法的c++代码
时间: 2024-03-06 17:50:29 浏览: 19
以下是一个简单的运用遗传算法求解TSP问题的C++代码:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
#include <random>
using namespace std;
// 定义城市坐标结构体
struct City {
double x;
double y;
};
// 随机生成城市坐标
vector<City> generate_cities(int n) {
vector<City> cities(n);
random_device rd;
mt19937 gen(rd());
uniform_real_distribution<> dis(0.0, 1.0);
for (int i = 0; i < n; i++) {
cities[i].x = dis(gen);
cities[i].y = dis(gen);
}
return cities;
}
// 计算两个城市之间的距离
double distance(City city1, City city2) {
double dx = city1.x - city2.x;
double dy = city1.y - city2.y;
return sqrt(dx*dx + dy*dy);
}
// 计算路径长度
double path_length(vector<int> path, vector<City> cities) {
double length = 0.0;
for (int i = 0; i < path.size() - 1; i++) {
length += distance(cities[path[i]], cities[path[i+1]]);
}
length += distance(cities[path.back()], cities[path.front()]);
return length;
}
// 交叉操作
vector<int> crossover(vector<int> parent1, vector<int> parent2) {
vector<int> child(parent1.size());
random_device rd;
mt19937 gen(rd());
uniform_int_distribution<> dis(0, parent1.size()-1);
int idx = dis(gen);
for (int i = 0; i < idx; i++) {
child[i] = parent1[i];
}
for (int i = idx; i < child.size(); i++) {
if (find(child.begin(), child.end(), parent2[i]) == child.end()) {
child[i] = parent2[i];
} else {
int j = 0;
while (find(child.begin(), child.end(), parent2[j]) != child.end()) {
j++;
}
child[i] = parent2[j];
}
}
return child;
}
// 变异操作
void mutation(vector<int>& path) {
random_device rd;
mt19937 gen(rd());
uniform_int_distribution<> dis(0, path.size()-1);
int idx1 = dis(gen);
int idx2 = dis(gen);
swap(path[idx1], path[idx2]);
}
int main() {
int n;
cout << "请输入城市数量:";
cin >> n;
vector<City> cities = generate_cities(n);
int population_size = 100;
vector<vector<int>> population(population_size, vector<int>(n));
for (int i = 0; i < population_size; i++) {
for (int j = 0; j < n; j++) {
population[i][j] = j;
}
random_shuffle(population[i].begin(), population[i].end());
}
int max_generations = 1000;
for (int i = 0; i < max_generations; i++) {
// 计算适应度
vector<double> fitness(population_size);
double total_fitness = 0.0;
for (int j = 0; j < population_size; j++) {
fitness[j] = 1.0 / path_length(population[j], cities);
total_fitness += fitness[j];
}
// 选择
vector<vector<int>> new_population(population_size, vector<int>(n));
for (int j = 0; j < population_size; j++) {
random_device rd;
mt19937 gen(rd());
uniform_real_distribution<> dis(0.0, total_fitness);
double r = dis(gen);
int k = 0;
while (r > 0) {
r -= fitness[k];
k++;
}
k--;
new_population[j] = population[k];
}
// 交叉
for (int j = 0; j < population_size / 2; j++) {
random_device rd;
mt19937 gen(rd());
uniform_real_distribution<> dis(0.0, 1.0);
double r = dis(gen);
if (r < 0.8) {
int idx1 = 2*j;
int idx2 = 2*j + 1;
vector<int> child1 = crossover(new_population[idx1], new_population[idx2]);
vector<int> child2 = crossover(new_population[idx2], new_population[idx1]);
new_population[idx1] = child1;
new_population[idx2] = child2;
}
}
// 变异
for (int j = 0; j < population_size; j++) {
random_device rd;
mt19937 gen(rd());
uniform_real_distribution<> dis(0.0, 1.0);
double r = dis(gen);
if (r < 0.1) {
mutation(new_population[j]);
}
}
population = new_population;
}
// 找到最优解
double min_length = path_length(population[0], cities);
int min_index = 0;
for (int i = 1; i < population_size; i++) {
double length = path_length(population[i], cities);
if (length < min_length) {
min_length = length;
min_index = i;
}
}
// 输出结果
cout << "最短路径为:";
for (int i = 0; i < n; i++) {
cout << population[min_index][i] << " ";
}
cout << "\n";
cout << "路径长度为:" << min_length << endl;
return 0;
}
```
该代码使用遗传算法求解TSP问题,首先随机生成城市坐标,然后生成初始种群,进行选择、交叉和变异等操作进行进化,并输出最短路径和路径长度。
相关推荐
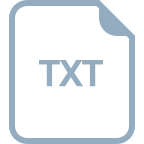
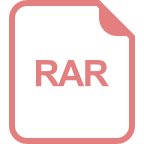
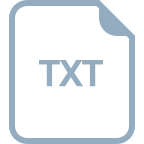















