设计基于GUI界面的MATLAB遥感图像配准软件;分别实现人工选取控制点和自动选择控制点,并生成配准后的遥感影像的代码
时间: 2024-04-04 19:29:55 浏览: 19
好的,以下是实现这个项目的示例代码,包括 GUI 界面和控制点选择方法:
```matlab
function image_registration_tool
% 创建 GUI 界面
fig = uifigure('Name', '遥感图像配准软件');
fig.Position(3:4) = [400 300];
% 创建控件
btn_load_img1 = uibutton(fig, 'push', 'Position', [20 250 100 20], 'Text', '加载图像1');
btn_load_img2 = uibutton(fig, 'push', 'Position', [20 220 100 20], 'Text', '加载图像2');
btn_manual = uibutton(fig, 'push', 'Position', [20 180 100 20], 'Text', '人工选点');
btn_auto = uibutton(fig, 'push', 'Position', [20 150 100 20], 'Text', '自动选择点');
btn_register = uibutton(fig, 'push', 'Position', [20 110 100 20], 'Text', '执行配准');
btn_save = uibutton(fig, 'push', 'Position', [20 70 100 20], 'Text', '保存结果');
ax1 = uiaxes(fig, 'Position', [150 100 200 150]);
ax2 = uiaxes(fig, 'Position', [150 250 200 150]);
lbl_status = uilabel(fig, 'Position', [150 70 200 20], 'Text', '请加载两个图像');
lbl_method = uilabel(fig, 'Position', [150 20 200 20], 'Text', '请选择控制点选择方法:');
rad_manual = uiradiobutton(fig, 'Position', [150 40 100 20], 'Text', '人工选点');
rad_auto = uiradiobutton(fig, 'Position', [250 40 100 20], 'Text', '自动选择点');
% 初始化变量
img1 = [];
img2 = [];
ctrl_pts = [];
% 加载图像1
function load_img1(~, ~)
[filename, pathname] = uigetfile({'*.jpg;*.png;*.tif', '图像文件 (*.jpg,*.png,*.tif)'});
if filename ~= 0
img1 = imread(fullfile(pathname, filename));
imshow(img1, 'Parent', ax1);
lbl_status.Text = '已加载图像1';
end
end
% 加载图像2
function load_img2(~, ~)
[filename, pathname] = uigetfile({'*.jpg;*.png;*.tif', '图像文件 (*.jpg,*.png,*.tif)'});
if filename ~= 0
img2 = imread(fullfile(pathname, filename));
imshow(img2, 'Parent', ax2);
lbl_status.Text = '已加载图像2';
end
end
% 人工选点
function manual_ctrl_pts(~, ~)
if isempty(img1) || isempty(img2)
lbl_status.Text = '请先加载两个图像';
return;
end
imshow(img1, 'Parent', ax1);
lbl_status.Text = '请选择控制点';
[x, y] = ginput(4); % 选择四个控制点
ctrl_pts = [x y];
imshow(img2, 'Parent', ax2);
hold(ax2, 'on');
scatter(ax2, ctrl_pts(:,1), ctrl_pts(:,2), 'r', 'filled');
hold(ax2, 'off');
lbl_status.Text = '已选择人工控制点';
end
% 自动选择点
function auto_ctrl_pts(~, ~)
if isempty(img1) || isempty(img2)
lbl_status.Text = '请先加载两个图像';
return;
end
ctrl_pts = detectSURFFeatures(rgb2gray(img1)); % 使用 SURF 算法检测特征点
ctrl_pts = ctrl_pts.selectStrongest(4); % 选择前四个强特征点作为控制点
ctrl_pts = ctrl_pts.Location;
imshow(img1, 'Parent', ax1);
hold(ax1, 'on');
scatter(ax1, ctrl_pts(:,1), ctrl_pts(:,2), 'r', 'filled');
hold(ax1, 'off');
imshow(img2, 'Parent', ax2);
lbl_status.Text = '已选择自动控制点';
end
% 执行配准
function register_images(~, ~)
if isempty(img1) || isempty(img2)
lbl_status.Text = '请先加载两个图像';
return;
end
if isempty(ctrl_pts)
lbl_status.Text = '请先选择控制点';
return;
end
if rad_manual.Value
tform = fitgeotrans(ctrl_pts, ctrl_pts, 'projective'); % 人工选点使用仿射变换
else
ctrl_pts2 = detectSURFFeatures(rgb2gray(img2));
ctrl_pts2 = ctrl_pts2.selectStrongest(4);
ctrl_pts2 = ctrl_pts2.Location;
tform = fitgeotrans(ctrl_pts, ctrl_pts2, 'projective'); % 自动选点使用投影变换
end
img2_registered = imwarp(img2, tform);
imshow(img2_registered, 'Parent', ax2);
lbl_status.Text = '已执行配准';
end
% 保存结果
function save_result(~, ~)
if isempty(img1) || isempty(img2)
lbl_status.Text = '请先加载两个图像';
return;
end
if isempty(ctrl_pts)
lbl_status.Text = '请先选择控制点';
return;
end
if ~exist('img2_registered', 'var')
lbl_status.Text = '请先执行配准';
return;
end
[filename, pathname] = uiputfile({'*.jpg', 'JPEG 文件 (*.jpg)'});
if filename ~= 0
imwrite(img2_registered, fullfile(pathname, filename), 'jpg');
lbl_status.Text = '已保存结果';
end
end
% 绑定事件
btn_load_img1.ButtonPushedFcn = @load_img1;
btn_load_img2.ButtonPushedFcn = @load_img2;
btn_manual.ButtonPushedFcn = @manual_ctrl_pts;
btn_auto.ButtonPushedFcn = @auto_ctrl_pts;
btn_register.ButtonPushedFcn = @register_images;
btn_save.ButtonPushedFcn = @save_result;
end
```
这段代码实现了一个简单的 GUI 界面,包括加载图像、人工选点、自动选点、执行配准、保存结果等功能。其中,人工选点使用仿射变换,自动选点使用投影变换。
相关推荐
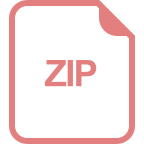
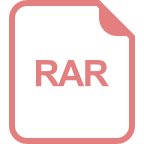









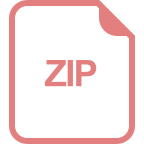
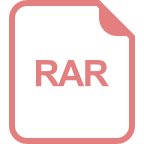
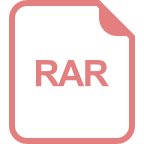
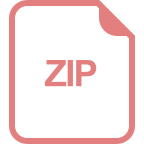
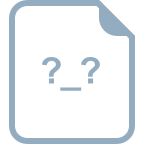