使用STM32l031xx的RTC定时器来计时30分钟,同时在接收到串口数据时通过一个标志位来记录有数据的情况,如果无数据则延时十秒重启
时间: 2024-02-01 07:13:07 浏览: 34
以下是使用STM32l031xx的RTC定时器来计时30分钟并处理串口数据的示例代码:
```c
#include "stm32l031xx.h"
#define RTC_WAKEUP_TIME_SEC 1800 // 30 minutes in seconds
volatile uint8_t data_received_flag = 0;
void USART1_IRQHandler(void)
{
if (USART1->ISR & USART_ISR_RXNE) {
USART1->RDR; // clear the RXNE flag by reading the received data
data_received_flag = 1;
}
}
void RTC_IRQHandler(void)
{
if (RTC->ISR & RTC_ISR_WUTF) {
RTC->ISR &= ~RTC_ISR_WUTF; // clear the wakeup timer interrupt flag
// do something when the wakeup timer expires
}
}
int main(void)
{
RCC->IOPENR |= RCC_IOPENR_GPIOAEN; // enable GPIOA clock
RCC->APB1ENR1 |= RCC_APB1ENR1_USART1EN; // enable USART1 clock
RCC->APB1ENR1 |= RCC_APB1ENR1_RTCEN; // enable RTC clock
// configure PA9 and PA10 as USART1 TX and RX pins
GPIOA->MODER &= ~(GPIO_MODER_MODE9_Msk | GPIO_MODER_MODE10_Msk);
GPIOA->MODER |= (GPIO_MODER_MODE9_1 | GPIO_MODER_MODE10_1);
GPIOA->AFR[1] |= (1 << (1 * 4)); // AF1 for PA9 (USART1_TX)
GPIOA->AFR[1] |= (1 << (2 * 4)); // AF1 for PA10 (USART1_RX)
// configure USART1
USART1->BRR = SystemCoreClock / 9600; // set baud rate to 9600
USART1->CR1 |= USART_CR1_RE | USART_CR1_RXNEIE; // enable RX and RXNE interrupt
// configure RTC wakeup timer
RTC->WUTR = RTC_WAKEUP_TIME_SEC - 1; // set wakeup time to 30 minutes
RTC->CR |= RTC_CR_WUTIE; // enable wakeup timer interrupt
RTC->CR &= ~RTC_CR_WUTE; // disable wakeup timer
RTC->ISR &= ~RTC_ISR_WUTF; // clear wakeup timer interrupt flag
RTC->PRER = (127 << RTC_PRER_PREDIV_A_Pos) | (255 << RTC_PRER_PREDIV_S_Pos); // set RTC clock to 1 Hz
RTC->CR |= RTC_CR_WUTE; // enable wakeup timer
NVIC_EnableIRQ(USART1_IRQn); // enable USART1 interrupt
NVIC_EnableIRQ(RTC_IRQn); // enable RTC interrupt
while (1) {
if (data_received_flag) {
data_received_flag = 0;
// process the received data
} else {
for (int i = 0; i < 1000000; i++) {} // delay for 1 second
RTC->CR &= ~RTC_CR_WUTE; // disable wakeup timer
RTC->ISR &= ~RTC_ISR_WUTF; // clear wakeup timer interrupt flag
RTC->WUTR = 10 - 1; // set wakeup time to 10 seconds
RTC->CR |= RTC_CR_WUTE; // enable wakeup timer
__WFI(); // enter sleep mode until next interrupt
}
}
}
```
在上面的代码中,我们首先启用了USART1和RTC的时钟,并将PA9和PA10配置为USART1的TX和RX引脚。然后,我们设置了USART1的波特率为9600,并启用了接收和接收中断。接下来,我们配置了RTC的唤醒定时器,将其设置为30分钟,并启用了唤醒定时器中断。在主循环中,我们检查数据接收标志位,如果有数据,则处理数据。否则,我们延迟10秒,然后将唤醒定时器设置为10秒,并进入睡眠模式等待下一个中断。当唤醒定时器中断发生时,我们会清除中断标志并执行相关操作。
相关推荐
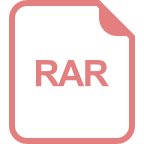
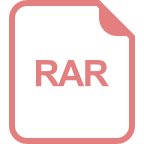
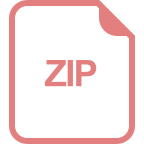
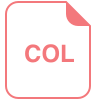
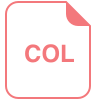
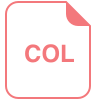
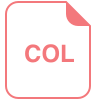
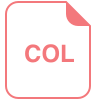









