stm32怎么用一个按键控制流水灯
时间: 2023-10-23 21:02:45 浏览: 343
要使用一个按键来控制流水灯,首先需要连接一个按键和一组LED灯到STM32开发板上。
首先,将按键的一端连接到STM32开发板的一个GPIO引脚上,另一端连接到地(GND)引脚上。确保按键连接正确,而且没有短路。
接下来,将一组LED灯连接到STM32开发板的几个GPIO引脚上。这些GPIO引脚将用于控制LED灯的亮灭。
在STM32的代码中,首先需要配置相应的GPIO引脚为输入和输出模式。可以使用STM32的库函数来实现这一步骤。
然后,需要编写一段代码来检测按键的状态。可以使用一个循环来不断地读取按键引脚的状态。如果按键被按下,即GPIO引脚的状态为高电平,则执行相应的操作。
一种简单的实现方式是使用一个变量来追踪当前LED灯的位置。可以使用一个循环移位操作来控制LED灯的流水效果。当按键被按下时,可以改变这个变量的值,从而改变LED灯的运行方向。
最后,需要在主循环中不断地更新LED灯的状态,以实现流水灯的效果。可以使用STM32的库函数来设置GPIO引脚的状态。
总结来说,要用一个按键控制流水灯,首先需要连接按键和LED灯到STM32开发板上,然后在代码中配置相应的GPIO引脚,并编写逻辑来检测按键状态和控制LED灯的流水效果。这样就可以通过按下按键来控制LED灯的亮灭和流水方向了。
相关问题
stm32一个按键控制8个流水灯
好的,这是一个比较简单的控制任务。你可以使用STM32的GPIO模块来实现按键和LED的控制。以下是一些实现步骤:
1. 首先,你需要初始化你的GPIO模块,以便可以控制LED和读取按键状态。你需要选择一个GPIO作为按键输入,以及8个GPIO用于控制LED输出。
2. 在主循环中,不断读取按键状态,并根据按键状态来改变LED的状态。例如,如果按键被按下,则将第一个LED点亮;如果再按一次,则将第一个LED熄灭并点亮第二个LED,以此类推。
3. 为了实现流水灯效果,你可以使用定时器中断或延时函数来控制LED的变化速度。在每个定时器中断或延时结束后,你需要将LED的状态更新为下一个。
这只是一个简单的实现思路,具体实现可能会因为硬件环境等因素而有所不同。
stm32f4一个按键控制8个流水灯变换
可以使用GPIO模块来控制按键和流水灯。首先需要配置GPIO为输入模式,然后轮询读取按键状态,如果检测到按键按下,则让流水灯进行变换。
以下是一个简单的代码实现:
```c
#include "stm32f4xx.h"
#define LED_GPIO_PORT GPIOD
#define LED_GPIO_PIN_0 GPIO_Pin_12
#define LED_GPIO_PIN_1 GPIO_Pin_13
#define LED_GPIO_PIN_2 GPIO_Pin_14
#define LED_GPIO_PIN_3 GPIO_Pin_15
#define BUTTON_GPIO_PORT GPIOA
#define BUTTON_GPIO_PIN GPIO_Pin_0
void Delay(__IO uint32_t nCount);
int main(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
uint32_t led_state = 0;
// 初始化GPIO时钟
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOD, ENABLE);
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
// 配置LED引脚为输出模式
GPIO_InitStructure.GPIO_Pin = LED_GPIO_PIN_0 | LED_GPIO_PIN_1 | LED_GPIO_PIN_2 | LED_GPIO_PIN_3;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(LED_GPIO_PORT, &GPIO_InitStructure);
// 配置按键引脚为输入模式
GPIO_InitStructure.GPIO_Pin = BUTTON_GPIO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_DOWN;
GPIO_Init(BUTTON_GPIO_PORT, &GPIO_InitStructure);
while (1)
{
// 检测按键状态
if (GPIO_ReadInputDataBit(BUTTON_GPIO_PORT, BUTTON_GPIO_PIN) == Bit_SET)
{
// 按键按下,改变LED状态
led_state = (led_state + 1) % 8;
// 控制LED流水灯
switch (led_state)
{
case 0:
GPIO_SetBits(LED_GPIO_PORT, LED_GPIO_PIN_0);
GPIO_ResetBits(LED_GPIO_PORT, LED_GPIO_PIN_1 | LED_GPIO_PIN_2 | LED_GPIO_PIN_3);
break;
case 1:
GPIO_SetBits(LED_GPIO_PORT, LED_GPIO_PIN_1);
GPIO_ResetBits(LED_GPIO_PORT, LED_GPIO_PIN_0 | LED_GPIO_PIN_2 | LED_GPIO_PIN_3);
break;
case 2:
GPIO_SetBits(LED_GPIO_PORT, LED_GPIO_PIN_2);
GPIO_ResetBits(LED_GPIO_PORT, LED_GPIO_PIN_0 | LED_GPIO_PIN_1 | LED_GPIO_PIN_3);
break;
case 3:
GPIO_SetBits(LED_GPIO_PORT, LED_GPIO_PIN_3);
GPIO_ResetBits(LED_GPIO_PORT, LED_GPIO_PIN_0 | LED_GPIO_PIN_1 | LED_GPIO_PIN_2);
break;
case 4:
GPIO_SetBits(LED_GPIO_PORT, LED_GPIO_PIN_0 | LED_GPIO_PIN_1);
GPIO_ResetBits(LED_GPIO_PORT, LED_GPIO_PIN_2 | LED_GPIO_PIN_3);
break;
case 5:
GPIO_SetBits(LED_GPIO_PORT, LED_GPIO_PIN_2 | LED_GPIO_PIN_3);
GPIO_ResetBits(LED_GPIO_PORT, LED_GPIO_PIN_0 | LED_GPIO_PIN_1);
break;
case 6:
GPIO_SetBits(LED_GPIO_PORT, LED_GPIO_PIN_0 | LED_GPIO_PIN_2);
GPIO_ResetBits(LED_GPIO_PORT, LED_GPIO_PIN_1 | LED_GPIO_PIN_3);
break;
case 7:
GPIO_SetBits(LED_GPIO_PORT, LED_GPIO_PIN_1 | LED_GPIO_PIN_3);
GPIO_ResetBits(LED_GPIO_PORT, LED_GPIO_PIN_0 | LED_GPIO_PIN_2);
break;
}
}
// 延时
Delay(1000000);
}
}
void Delay(__IO uint32_t nCount)
{
while (nCount--)
{
}
}
```
在这个代码中,我们使用了GPIO模块来控制LED流水灯和按键。首先,我们需要初始化GPIO时钟,并将LED引脚配置为输出模式,按键引脚配置为输入模式。在主循环中,我们轮询读取按键状态,如果检测到按键按下,则改变LED流水灯的状态。具体来说,我们通过一个变量来记录LED的状态,并使用switch语句来控制LED引脚的电平。最后,我们添加了一个简单的延时函数以减缓LED变换的速度。
需要注意的是,由于本代码使用的是轮询方式读取按键状态,因此需要添加适当的去抖动措施以避免误触发。另外,如需加入更多的功能,需要对代码进行相应的修改。
相关推荐
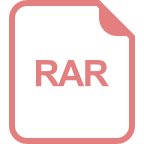
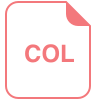
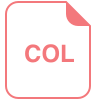
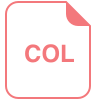
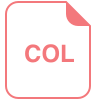
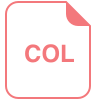







