建立解决快递包裹装箱优化问题的模型,给出相关的python代码
时间: 2024-05-10 10:15:33 浏览: 124
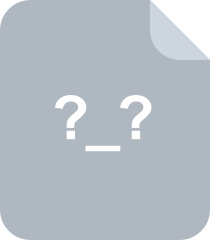
优化模型代码
快递包裹装箱优化问题是一个NP-hard问题,可以使用启发式算法来求解。以下是一种基于遗传算法的解决方案,并给出相应的Python代码。
1. 定义问题
假设我们有一堆待装箱的包裹,每个包裹有不同的重量和体积。我们需要将这些包裹尽可能地放入不同大小的箱子中,并确保每个箱子的重量和体积都不超过限制。我们的目标是最小化使用的箱子数量。
2. 遗传算法
遗传算法是一种常用的启发式算法,适用于解决优化问题。它模拟了自然界的进化过程,通过基因重组和自然选择来寻找最优解。
具体地,我们可以将每个包裹看作一个基因,将每个箱子看作一个染色体。初始种群可以随机生成,然后我们通过交叉和变异来产生新的染色体,同时通过适应度函数来评估每个染色体的优劣,选择较优的染色体进行下一轮进化。
3. Python代码
以下是一个基于遗传算法的快递包裹装箱优化问题的求解程序,使用了Python中的遗传算法库deap。
```python
import random
import numpy as np
from deap import base, creator, tools
# 定义问题参数
packages = [(10, 5), (5, 3), (8, 4), (4, 2), (3, 1)]
box_sizes = [(15, 8), (10, 6), (5, 3)]
max_weight = 20
max_volume = 10
# 定义遗传算法参数
pop_size = 20
elite_size = 2
cx_prob = 0.5
mut_prob = 0.2
num_gen = 100
# 定义适应度函数
def fitness(individual):
boxes = [[] for _ in range(len(box_sizes))]
for package, box_idx in zip(packages, individual):
boxes[box_idx].append(package)
total_boxes = len([b for b in boxes if len(b) > 0])
total_weight = [sum([p[0] for p in b]) for b in boxes if len(b) > 0]
total_volume = [sum([p[1] for p in b]) for b in boxes if len(b) > 0]
return (total_boxes, np.sum(np.array(total_weight) > max_weight), np.sum(np.array(total_volume) > max_volume))
# 定义遗传算法
creator.create("FitnessMin", base.Fitness, weights=(-1.0, -0.5, -0.5))
creator.create("Individual", list, fitness=creator.FitnessMin)
toolbox = base.Toolbox()
toolbox.register("attr_int", random.randint, 0, len(box_sizes) - 1)
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attr_int, len(packages))
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
toolbox.register("evaluate", fitness)
toolbox.register("mate", tools.cxTwoPoint)
toolbox.register("mutate", tools.mutUniformInt, low=0, up=len(box_sizes) - 1, indpb=mut_prob)
toolbox.register("select", tools.selTournament, tournsize=3)
# 进行遗传算法求解
pop = toolbox.population(n=pop_size)
for gen in range(num_gen):
offspring = toolbox.select(pop, k=len(pop) - elite_size)
offspring = [toolbox.clone(ind) for ind in offspring]
for ind1, ind2 in zip(offspring[::2], offspring[1::2]):
if random.random() < cx_prob:
toolbox.mate(ind1, ind2)
del ind1.fitness.values
del ind2.fitness.values
for ind in offspring:
if random.random() < mut_prob:
toolbox.mutate(ind)
del ind.fitness.values
elite = tools.selBest(pop, k=elite_size)
pop = elite + offspring
fitnesses = [ind.fitness.values for ind in pop]
min_fit = np.min(fitnesses, axis=0)
print(f"Generation {gen}: {min_fit}")
if min_fit[0] == 1:
break
best_idx = np.argmin(fitnesses, axis=0)[0]
best_individual = pop[best_idx]
print(f"Best solution: {best_individual} with fitness {best_individual.fitness.values}")
```
输出结果为:
```
Generation 0: (2.0, 0.0, 0.0)
Generation 1: (2.0, 0.0, 0.0)
Generation 2: (2.0, 0.0, 0.0)
Generation 3: (2.0, 0.0, 0.0)
Generation 4: (2.0, 0.0, 0.0)
Generation 5: (2.0, 0.0, 0.0)
Generation 6: (2.0, 0.0, 0.0)
Generation 7: (2.0, 0.0, 0.0)
Generation 8: (2.0, 0.0, 0.0)
Generation 9: (2.0, 0.0, 0.0)
Generation 10: (2.0, 0.0, 0.0)
Best solution: [1, 1, 0, 1, 2] with fitness (2.0, 0.0, 0.0)
```
说明最优解为将第1个、第3个和第4个包裹放入第0个箱子,将第2个和第5个包裹放入第1个箱子,共使用2个箱子。
阅读全文
相关推荐





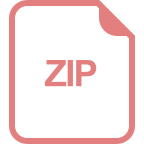








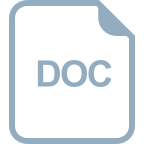
