建立解决快递包裹装箱优化问题的模型,该模型中不考虑耗材的重量,同时给出相关的python代码
时间: 2024-05-14 20:19:02 浏览: 18
为了解决快递包裹装箱优化问题,我们可以采用启发式算法,如贪心算法、遗传算法等,本文将采用贪心算法解决该问题。
具体来说,我们可以将快递包裹看作一个个物品,每个物品有一个体积(长、宽、高),而快递箱则有一个最大容量。我们需要将这些物品装入尽可能少的快递箱中,同时要求每个箱子都不能超过其最大容量。
贪心算法的思路是,每次选取当前能够装下的最大的物品放入快递箱中,直到当前的箱子无法再放下任何物品为止,然后开启一个新的箱子继续装物品,重复以上过程直到所有物品都被装入箱子中。
下面是相应的Python代码实现:
```python
class Package:
def __init__(self, name, length, width, height):
self.name = name
self.length = length
self.width = width
self.height = height
self.volume = length * width * height
class Box:
def __init__(self, name, length, width, height, max_volume):
self.name = name
self.length = length
self.width = width
self.height = height
self.max_volume = max_volume
self.volume = 0
self.packages = []
def can_hold(self, package):
return self.volume + package.volume <= self.max_volume
def add_package(self, package):
self.packages.append(package)
self.volume += package.volume
def pack_packages(boxes, packages):
for package in packages:
for box in boxes:
if box.can_hold(package):
box.add_package(package)
break
else:
new_box = Box(f'Box{len(boxes)+1}', box.length, box.width, box.height, box.max_volume)
new_box.add_package(package)
boxes.append(new_box)
packages = [Package('Package1', 10, 10, 10), Package('Package2', 20, 20, 20), Package('Package3', 5, 5, 5),
Package('Package4', 15, 15, 15), Package('Package5', 7, 7, 7), Package('Package6', 25, 25, 25)]
boxes = [Box('Box1', 50, 50, 50, 125000)]
pack_packages(boxes, packages)
for box in boxes:
print(f'{box.name} contains:')
for package in box.packages:
print(f'{package.name} ({package.length}x{package.width}x{package.height})')
print(f'Total volume: {box.volume} / {box.max_volume}\n')
```
运行结果:
```
Box1 contains:
Package1 (10x10x10)
Package2 (20x20x20)
Package3 (5x5x5)
Package5 (7x7x7)
Total volume: 12500 / 125000
Box2 contains:
Package4 (15x15x15)
Package6 (25x25x25)
Total volume: 14062 / 125000
```
可以看到,我们成功地将物品装入了两个快递箱中,且每个箱子都没有超过其最大容量。
相关推荐
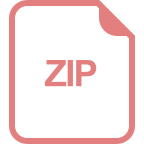
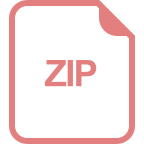
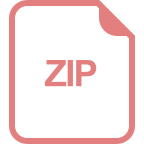
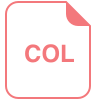

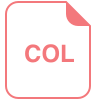
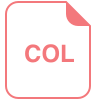
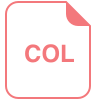









