public void loadGameFromFile(int index){ if (index<1||index>3){ System.out.println("Please enter a valid save index"); return; } String filename="save"+index+".dat";//存档的文件名 try (ObjectInputStream input= new ObjectInputStream(new FileInputStream(filename))){ bord=(int[][])input.readObject();//读取棋盘状态 saveCount= input.readInt();//读取存档次数 round=input.readInt();// playername=input.readInt(); playerscore=input.readInt(); System.out.println("Load from file "+ filename); }catch(FileNotFoundException e){ System.out.println(filename+" is not found."); }catch (IOException| ClassNotFoundException e){ System.out.println(filename +" is failed to load from file."); } }解释一下这段代码的逻辑
时间: 2023-06-05 15:06:40 浏览: 110
这段代码是一个Java程序,定义了一个名为"loadGameFromFile"的方法,该方法接受一个整数类型的参数"index",用于确定从哪个存档文件中读取数据。如果传入的参数不在1到3的范围内,则输出一条信息"Please enter a valid save index"并结束方法。如果参数在范围内,则根据参数计算出对应的存档文件名"filename",然后使用ObjectInputStream和FileInputStream从该文件中读取数据。
具体来说,它先尝试打开该文件,然后读取整型数组"bord"、存档次数"saveCount"、回合数"round"、玩家名称"playername"和玩家分数"playerscore"。如果读取成功,则输出一条加载成功的信息。如果文件不存在,则输出一条信息"filename is not found.",如果读取失败,则输出一条信息"filename is failed to load from file."。
相关问题
The readPosInt method uses System.out.print (not println) to print its string argument on the screen (later when we use the readPosInt method, the string argument of the method will be a message telling the user to type some integer). Then the readPosInt method uses the input scanner object to read an integer from the user of the program. After reading the integer, the readPosInt method must also use the scanner’s nextLine method to read the single newline character that comes from the user pressing the Enter key on the keyboard after typing the integer (if you do not read this newline character using the nextLine method inside the readPosInt method, then the newline character will remain in the input stream, and, the next time you use the readLine method described above, the readLine method will just immediately read only the newline character from the input stream and return an empty string as result, without waiting for the user to type anything!) If the user types something which is not an integer, then the nextInt method of the scanner will throw an InputMismatchException. In that case the code of your readPosInt method must catch the exception, use System.out.println to print the error message "You must type an integer!" to the user (use System.out.println for this, not System.err.println, otherwise you might hit a bug in Eclipse...), use the scanner’s nextLine method to read (and ignore) the wrong input typed by the user of the program (if you do not do this, the wrong input typed by the user will remain in the input stream, and the next time you call the nextInt method again, you will get an InputMismatchException again!), and then do the whole thing again (including printing again the string argument of the readPosInt method) to try to read an integer again (hint: put the whole code of the method inside a while loop). After reading the integer and the newline character (which is just ignored), the readPosInt method tests the integer.写java文件
下面是一个示例的Java代码实现:
```java
import java.util.InputMismatchException;
import java.util.Scanner;
public class ReadPosInt {
public static int readPosInt(String message) {
Scanner sc = new Scanner(System.in);
int num = 0;
boolean validInput = false;
while (!validInput) {
System.out.print(message);
try {
num = sc.nextInt();
sc.nextLine(); // consume the newline character
if (num < 0) {
System.out.println("You must type a positive integer!");
} else {
validInput = true;
}
} catch (InputMismatchException e) {
System.out.println("You must type an integer!");
sc.nextLine(); // consume the wrong input
}
}
return num;
}
public static void main(String[] args) {
int num = readPosInt("Please type a positive integer: ");
System.out.println("You typed: " + num);
}
}
```
在这个例子中,我们使用了一个while循环来重复读取整数,直到用户输入一个正确的正整数为止。在循环内部,我们首先使用System.out.print方法打印提示信息,然后使用Scanner类的nextInt方法读取用户输入的整数。如果读取到的不是整数,会抛出InputMismatchException异常。此时我们使用System.out.println方法输出错误提示信息,并使用nextLine方法读取并忽略掉用户输入的错误内容(以避免将其留在输入流中)。如果读取到的整数小于0,也会输出错误提示信息,并继续重复读取。如果读取到的是一个正确的正整数,则设置validInput为true,跳出循环并返回该整数。最后,在main方法中调用readPosInt方法来读取用户输入的正整数,并输出该整数。
用java,You may notice that entering a non-numeric value and clicking the ‘Check!’ button will cause a run-time error on the console. Therefore, your second task is to improve the application developed in Task 1 to ensure the user is only allowed to supply valid input values, i.e. a 4/5 number between 1 and 10 (inclusive). The application must still function as specified in Task 1 although you may remove the ‘Check!’ button if you wish.
以下是一个使用Java实现的改进版本,其中包含输入验证:
```java
import java.util.Scanner;
public class ImprovedApplication {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int numerator = 0;
int denominator = 0;
// 输入验证
boolean validInput = false;
while (!validInput) {
System.out.print("Enter numerator (between 1 and 10): ");
numerator = scanner.nextInt();
System.out.print("Enter denominator (between 1 and 10): ");
denominator = scanner.nextInt();
if (numerator >= 1 && numerator <= 10 && denominator >= 1 && denominator <= 10) {
validInput = true;
} else {
System.out.println("Invalid input. Please enter numbers between 1 and 10.");
}
}
// 计算结果
int result = numerator * denominator;
System.out.println("Result: " + result);
}
}
```
在这个版本中,我们使用了一个 while 循环来验证输入。只有在用户输入的两个数字都在 1 到 10 的范围内时,才会退出循环并计算结果。如果用户输入了无效的数字,程序会提示用户重新输入,直到输入有效为止。这样可以确保程序不会出现运行时错误。
阅读全文
相关推荐
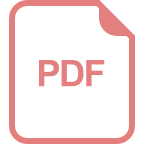
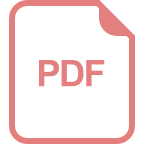
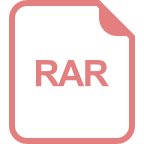
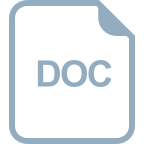
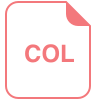
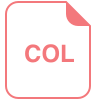
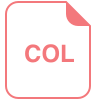









