来一段Verilog的case语句
时间: 2023-12-08 15:20:03 浏览: 75
以下是一个简单的Verilog的case语句的例子:
```
module example_case (
input [1:0] sel,
output reg [3:0] out
);
always @ (*)
begin
case (sel)
2'b00: out = 4'b0000;
2'b01: out = 4'b0001;
2'b10: out = 4'b0010;
2'b11: out = 4'b0011;
default: out = 4'bXXXX; // 如果sel的值不是00、01、10或11,则out的值为未定义
endcase
end
endmodule
```
在上述代码中,case语句的输入是sel,它是一个2位的输入端口。case语句中的每个分支都对应于一个特定的sel值,并且每个分支都有一个对应的out值。当sel值等于某个分支时,out值将被设置为对应的值。如果sel的值不是00、01、10或11,则out的值为未定义。
相关问题
verilog case语句
Verilog是一种硬件描述语言(HDL),用于设计和描述数字电路和系统。`case`语句在Verilog中是一个重要的控制结构,它允许根据给定的一组条件来选择执行哪一段代码块。它的基本语法如下:
```verilog
case(expression)
case_value_1 : statement_1;
case_value_2 : statement_2;
...
default : statement_default; // 可选,默认情况
endcase
```
在这里,`expression`是一个布尔或整数值,`case_value`是一系列可能的匹配值,每个`case_value`后面跟着对应的`statement`,如果`expression`的值等于某个`case_value`,那么相应的`statement`就会执行。
例如:
```verilog
reg input;
wire output;
always @(input) begin
case(input)
0 : output = 0;
1 : output = 1;
2 : output = input << 1;
default : output = -1; // 默认情况
endcase
end
```
在这个例子中,`output`的值会根据`input`的不同取值动态改变。
Verilog语言case语句和if else语句
Verilog是一种硬件描述语言,用于设计和验证数字系统的行为。它支持`case`语句和`if-else`语句作为控制流的关键元素。
`case`语句允许你基于一个输入信号的值执行一系列条件分支。它的基本结构如下:
```verilog
case (input_variable)
case_value1 : // 当 input_variable 等于 case_value1 时执行这里的代码
case_value2 : // 类似地,每个case_value对应一段代码块
default : // 如果 input_variable 没匹配到前面的值,则执行default分支
endcase
```
`if-else`语句则是标准的条件判断结构,类似于大多数编程语言:
```verilog
if (condition) begin
// 如果 condition 成立,执行这里的代码
code_block;
end
else begin
// 否则(即 condition 不成立),执行这里的代码
other_code_block;
end
```
两者的主要区别在于灵活性:`case`更适合处理离散的、有限数量的选择情况,而`if-else`更适用于复杂的条件判断或多路分支的情况。然而,`case`通常可以简化代码,并通过`default`提供默认操作。
阅读全文
相关推荐
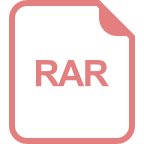
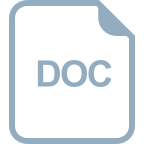
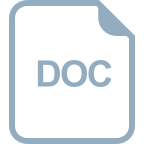
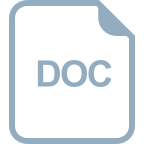
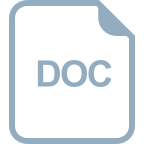
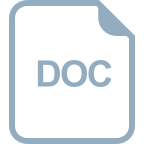







