将古典密码算法、对称密码算法、公钥密码算法和单向散列算法的实现附带源代码
时间: 2024-07-28 18:00:36 浏览: 56
密码学中的算法种类繁多,包括古典密码、对称密钥加密、非对称密钥加密(公钥)以及单向散列函数。由于这些算法的具体实现涉及大量代码,并且源代码可能因编程语言和库的不同而有所差异,这里我将提供一个概述,但不会直接给出完整的源代码。
1. **古典密码**:如Caesar Cipher(凯撒密码)和Vigenère Cipher(维吉尼亚密码),它们通常手动实现,没有现代加密库中的复杂性和安全性。在Python中,可以这样简单实现Caesar Cipher:
```python
def caesar_cipher(text, shift):
encrypted_text = ""
for char in text:
if char.isalpha():
shift_amount = shift % 26
if char.islower():
new_char = chr((ord(char) - ord('a') + shift_amount) % 26 + ord('a'))
else:
new_char = chr((ord(char) - ord('A') + shift_amount) % 26 + ord('A'))
encrypted_text += new_char
else:
encrypted_text += char
return encrypted_text
```
2. **对称密钥加密**:如AES(高级加密标准)使用的是相同的密钥进行加密和解密。你可以使用像PyCryptodome这样的库,但它包含了大量的代码:
```python
from Crypto.Cipher import AES
key = b'Sixteen byte key'
cipher = AES.new(key, AES.MODE_ECB)
plaintext = b'This is a secret message'
ciphertext = cipher.encrypt(plaintext)
```
3. **公钥密码算法**(如RSA)涉及到一对密钥:公钥用于加密,私钥用于解密。Python中的`cryptography`库可以实现这个:
```python
from cryptography.hazmat.primitives.asymmetric import rsa, padding
from cryptography.hazmat.primitives import serialization
key = rsa.generate_private_key(public_exponent=65537, key_size=2048)
public_key = key.public_key()
message = b'This is an encrypted message'
encrypted_message = public_key.encrypt(message, padding.OAEP(mgf=padding.MGF1(algorithm=hashes.SHA256()), algorithm=hashes.SHA256(), label=None))
```
4. **单向散列函数**(如SHA-256)用于数据完整性检查,不提供可逆的加密。Python的`hashlib`模块有实现:
```python
import hashlib
hash_object = hashlib.sha256(b'This is a message')
hash_value = hash_object.hexdigest()
```
阅读全文
相关推荐
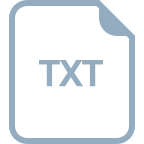
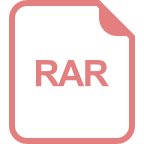
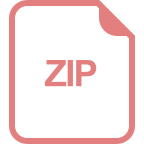















