模拟退火算法求Rosenbrock
时间: 2023-06-21 08:11:44 浏览: 44
Rosenbrock函数是一个经典的非凸优化问题,其函数形式为:
f(x,y) = (1-x)^2 + 100(y-x^2)^2
其中,x和y是变量。
下面介绍如何使用模拟退火算法求解Rosenbrock函数的最小值。
1. 定义初始状态
首先需要定义初始状态。可以随机生成一个初始状态,即随机生成x和y的值。例如,假设x和y的取值范围均为[-5,5],则可以使用如下代码生成一个初始状态:
```
import random
x = random.uniform(-5, 5)
y = random.uniform(-5, 5)
state = (x, y)
```
2. 定义能量函数
接下来需要定义能量函数。Rosenbrock函数就是我们要求解的能量函数。可以使用如下代码定义Rosenbrock函数:
```
def rosenbrock(x, y):
return (1 - x)**2 + 100 * (y - x**2)**2
```
3. 定义邻域函数
模拟退火算法需要定义邻域函数,用于生成新的状态。可以使用如下代码定义邻域函数:
```
def neighbor(state):
x, y = state
dx = random.uniform(-0.5, 0.5)
dy = random.uniform(-0.5, 0.5)
return (x+dx, y+dy)
```
该邻域函数会在当前状态的基础上,随机生成一个小的偏移量,生成新的状态。
4. 定义温度函数
模拟退火算法需要定义温度函数,用于控制搜索过程中的温度变化。可以使用如下代码定义温度函数:
```
def temperature(step):
return max(0.01, 1 - step/1000)
```
该温度函数会随着搜索步数的增加而降低温度。
5. 定义搜索函数
最后,可以定义搜索函数,使用模拟退火算法搜索Rosenbrock函数的最小值。可以使用如下代码定义搜索函数:
```
def simulated_annealing(start_state, energy_func, neighbor_func, temperature_func):
state = start_state
energy = energy_func(*state)
for step in range(10000):
new_state = neighbor_func(state)
new_energy = energy_func(*new_state)
delta_energy = new_energy - energy
if delta_energy < 0 or random.random() < math.exp(-delta_energy/temperature_func(step)):
state = new_state
energy = new_energy
return state, energy
```
该搜索函数会在初始状态的基础上,不断地生成新的状态,并计算每个状态的能量值。如果新状态的能量值更低,那么就接受该状态;否则,以一定的概率接受该状态。随着搜索步数的增加,温度会降低,使得接受劣解的概率逐渐降低。
6. 运行搜索函数
最后,可以运行搜索函数,求解Rosenbrock函数的最小值。可以使用如下代码运行搜索函数:
```
import math
start_state = (0, 0)
final_state, final_energy = simulated_annealing(start_state, rosenbrock, neighbor, temperature)
print("Final state:", final_state)
print("Final energy:", final_energy)
```
该代码会输出最小值的状态和能量值。
相关推荐
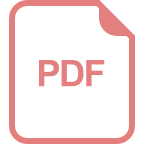







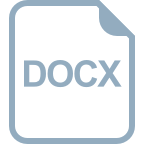
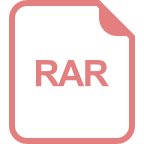
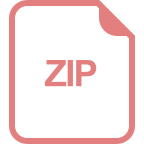
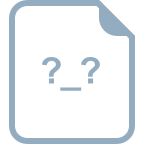
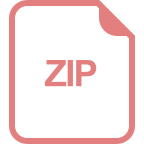
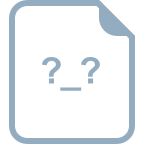