python输入一个正整数n,将文件中的文本读取为字符串,利用jieba库的精确模式将文本切分为字符串,并输出前n个字符串的列表
时间: 2024-02-12 19:06:25 浏览: 29
好的,我理解了你的要求。以下是实现这个功能的 Python 代码:
```python
import jieba
# 读取文件内容为字符串
with open('file.txt', 'r', encoding='utf-8') as f:
content = f.read()
# 利用jieba库的精确模式将文本切分为字符串
word_list = jieba.lcut(content)
# 输出前n个字符串的列表
n = int(input("请输入一个正整数n:"))
print(word_list[:n])
```
这个代码中,我们首先使用 Python 的内置函数 `open()` 读取文件内容为字符串,并存储在变量 `content` 中。接着,我们调用 `jieba.lcut()` 函数,将 `content` 字符串按照中文分词的方式切分为字符串列表 `word_list`。最后,我们根据用户输入的正整数 `n`,使用 Python 的列表切片操作 `word_list[:n]` 输出前 `n` 个字符串的列表。
请注意,这个代码中读取文件的文件名为 `file.txt`,你需要将其替换为你实际使用的文件名。另外,jieba库需要提前安装,你可以使用 `pip install jieba` 命令进行安装。
相关问题
python输入一个正整数n,将文件中的文本读取为字符串,利用jieba库的精确模式将文本切分为字符串,统计每个字符串出现的频率,存储为字典类型,输出词频最高的元素为二元组的列表前n项
好的,我理解了你的问题。下面是对应的 Python 代码:
```python
import jieba
# 读取文件内容为字符串
with open('file.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 利用 jieba 库的精确模式进行分词
words = list(jieba.cut(text))
# 统计每个字符串出现的频率
freq_dict = {}
for w in words:
if w not in freq_dict:
freq_dict[w] = 1
else:
freq_dict[w] += 1
# 对字典按照值进行排序,得到词频最高的元素为二元组的列表
freq_list = sorted(freq_dict.items(), key=lambda x: x[1], reverse=True)
# 输出前n项
n = int(input("请输入n的值:"))
for i in range(n):
print(freq_list[i])
```
请注意,上述代码中的 `file.txt` 应该改为你实际的文件名。同时,你需要安装 jieba 库,可以使用以下命令进行安装:
```
pip install jieba
```
如果你在使用过程中遇到问题,可以随时向我提问。
python输入一个正整数n,将文件中的文本读取为字符串,利用jieba库的精确模式将文本切分为字符串,将无实质意义的单字符元素去掉,统计长度大于1的每个字符串出现的频率,存储为字典类型,输出词频最高的元素为二元组的列表前n项。
好的,这是一个比较复杂的问题,需要分步骤来解决。首先,你需要安装jieba库,可以使用以下命令进行安装:
```
pip install jieba
```
接下来,你可以使用以下代码读取文件并进行分词:
```python
import jieba
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as f:
text = f.read()
return text
def cut_words(text):
words = jieba.cut(text, cut_all=False)
return [word for word in words if len(word) > 1]
text = read_file('example.txt')
words = cut_words(text)
```
这段代码中,`read_file()`函数可以读取文件,`cut_words()`函数使用jieba库进行分词,并过滤掉长度小于等于1的单字符元素。
接下来,你需要统计每个字符串出现的频率,并存储为字典类型。可以使用以下代码完成这个任务:
```python
def count_words(words):
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
return word_count
word_count = count_words(words)
```
这段代码中,`count_words()`函数使用字典类型`word_count`来存储每个字符串出现的频率。如果一个字符串已经在字典中出现过,就将其频率加1;否则,将该字符串添加到字典中,并将其频率设为1。
最后,你需要输出词频最高的元素为二元组的列表前n项。可以使用以下代码完成这个任务:
```python
def top_n_words(word_count, n):
sorted_words = sorted(word_count.items(), key=lambda x:x[1], reverse=True)
return sorted_words[:n]
top_n = top_n_words(word_count, n)
print(top_n)
```
这段代码中,`top_n_words()`函数使用`sorted()`函数将字典中的元素按照频率从高到低排序,并返回前n项。最后,你可以使用`print()`函数输出结果。
完整代码如下:
```python
import jieba
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as f:
text = f.read()
return text
def cut_words(text):
words = jieba.cut(text, cut_all=False)
return [word for word in words if len(word) > 1]
def count_words(words):
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
return word_count
def top_n_words(word_count, n):
sorted_words = sorted(word_count.items(), key=lambda x:x[1], reverse=True)
return sorted_words[:n]
text = read_file('example.txt')
words = cut_words(text)
word_count = count_words(words)
top_n = top_n_words(word_count, n)
print(top_n)
```
相关推荐
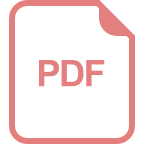
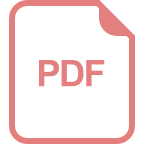












