将文件中的文本读取为字符串,利用jieba库的精确模式将文本切分为字符串,将无实质意义的单字符元素去掉,统计长度大于1的每个字符串出现的频率,存储为字典类型,输出词频最高的元素为二元组的列表前n项。
时间: 2024-02-18 08:03:19 浏览: 115
好的,我明白了你的问题。以下是解决方案:
```python
import jieba
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as f:
text = f.read()
return text
def cut_text(text):
words = list(jieba.cut(text))
result = []
for w in words:
if len(w) > 1:
result.append(w)
return result
def get_word_frequency(text, n):
word_dict = {}
for w in cut_text(text):
if w in word_dict:
word_dict[w] += 1
else:
word_dict[w] = 1
sorted_words = sorted(word_dict.items(), key=lambda x: x[1], reverse=True)
return sorted_words[:n]
file_path = 'example.txt'
text = read_file(file_path)
result = get_word_frequency(text, 10)
print(result)
```
解释一下以上代码的每个步骤:
1. `read_file(file_path)` 函数用于读取文件中的文本,并返回一个字符串。
2. `cut_text(text)` 函数接收一个字符串作为参数,使用 `jieba` 库将文本切分为字符串,并去掉长度为1的单字符元素,返回一个列表。
3. `get_word_frequency(text, n)` 函数接收一个字符串和一个整数作为参数,统计长度大于1的每个字符串出现的频率,返回一个按词频从高到低排序的二元组(元组的第一个元素为字符串,第二个元素为词频)列表,其中只包含前n项。
4. 最后调用函数,将结果输出。
注意:以上代码中使用的是 `jieba` 库的精确模式,如果需要使用其他模式,可以在 `jieba.cut()` 函数中传入 `cut_all=True` 或 `cut_for_search=True` 参数。同时,以上代码中的文件路径为相对路径,如果需要使用绝对路径,请自行修改。
阅读全文
相关推荐
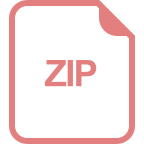















