用C语言编写代码,删除一个队列中的一个指定元素e并写出完整代码
时间: 2023-06-08 15:04:21 浏览: 70
好的,以下是用C语言编写代码,删除一个队列中的一个指定元素e的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#define MAX_QUEUE_SIZE 100
typedef struct {
int front; // 队头指针
int rear; // 队尾指针
int queue[MAX_QUEUE_SIZE]; // 队列数组
} Queue;
// 初始化队列
void initQueue(Queue *q) {
q->front = 0;
q->rear = 0;
}
// 判断队列是否为空
int isEmpty(Queue *q) {
return (q->front == q->rear);
}
// 判断队列是否已满
int isFull(Queue *q) {
return ((q->rear + 1) % MAX_QUEUE_SIZE == q->front);
}
// 将元素e入队
void enqueue(Queue *q, int e) {
if (isFull(q)) {
printf("Queue is full\n");
return;
}
q->queue[q->rear] = e;
q->rear = (q->rear + 1) % MAX_QUEUE_SIZE;
}
// 删除队头元素并返回其值
int dequeue(Queue *q) {
if (isEmpty(q)) {
printf("Queue is empty\n");
return -1;
}
int e = q->queue[q->front];
q->front = (q->front + 1) % MAX_QUEUE_SIZE;
return e;
}
// 删除指定元素
void deleteElement(Queue *q, int e) {
int count = 0; // 统计删除的元素个数
int temp[MAX_QUEUE_SIZE]; // 临时数组
while (!isEmpty(q)) {
int front = dequeue(q);
if (front != e) { // 如果队头元素不是指定元素,则加入临时数组
temp[count++] = front;
}
}
for (int i = 0; i < count; i++) { // 将临时数组中的元素重新加入队列
enqueue(q, temp[i]);
}
}
int main() {
Queue q;
initQueue(&q);
enqueue(&q, 1);
enqueue(&q, 2);
enqueue(&q, 3);
enqueue(&q, 4);
enqueue(&q, 5);
deleteElement(&q, 3); // 删除指定元素3
while (!isEmpty(&q)) {
printf("%d ", dequeue(&q));
}
printf("\n");
return 0;
}
```
以上代码通过循环遍历队列,将队头元素不是指定元素的元素加入临时数组中,最后将临时数组中的元素重新加入队列,实现了删除队列中的一个指定元素的功能。
相关推荐
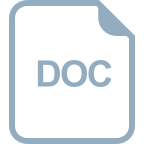
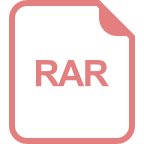
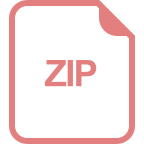
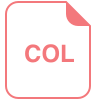
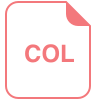
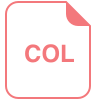
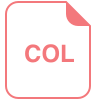
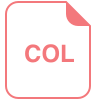









