使用C++实现菜谱生成的代码
时间: 2024-02-19 19:02:56 浏览: 29
要使用C++实现菜谱生成的代码,可以采用以下步骤:
1. 定义菜谱和食材数据结构,如:
```
struct Recipe {
string name;
vector<string> ingredients;
string instructions;
};
struct Ingredient {
string name;
int quantity;
string brand;
};
```
2. 读取菜谱和食材数据,可以从文件或数据库中读取。
3. 对用户输入的食材进行处理,提取关键词和特征,如:
```
vector<string> extractKeywords(string input) {
// todo: 实现关键词提取算法
return keywords;
}
```
4. 根据用户输入的食材预测可能的菜品,可以使用机器学习模型或基于规则的匹配算法,如:
```
vector<Recipe> predictRecipes(vector<string> keywords, vector<Recipe> recipes) {
vector<Recipe> result;
for (Recipe recipe : recipes) {
bool match = true;
for (string keyword : keywords) {
if (find(recipe.ingredients.begin(), recipe.ingredients.end(), keyword) == recipe.ingredients.end()) {
match = false;
break;
}
}
if (match) {
result.push_back(recipe);
}
}
return result;
}
```
5. 返回匹配的菜品给用户,如:
```
void printRecipes(vector<Recipe> recipes) {
for (Recipe recipe : recipes) {
cout << recipe.name << endl;
cout << "Ingredients: ";
for (string ingredient : recipe.ingredients) {
cout << ingredient << " ";
}
cout << endl;
cout << "Instructions: " << recipe.instructions << endl;
}
}
```
以上是一个简单的菜谱生成的代码示例,具体实现还需要根据具体的需求和数据结构进行调整和完善。同时,还需要考虑输入数据的安全性和用户体验问题,如输入校验、结果排序等。
相关推荐
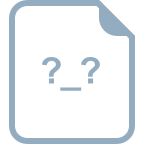
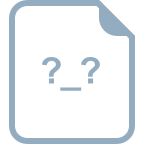














