C++实现CSV生成折线图
时间: 2023-07-11 19:33:16 浏览: 190
要实现CSV生成折线图,可以使用C++中的第三方库来完成。
其中,我推荐使用Qt框架中的QCustomPlot库。QCustomPlot是一个基于Qt的开源图形库,可以实现多种类型的图形绘制,包括折线图、柱状图、散点图等。
以下是实现CSV生成折线图的大致步骤:
1. 读取CSV文件,将数据存储到二维数组中。
2. 使用QCustomPlot库绘制折线图。可以使用QCustomPlot的QCPGraph类来绘制折线图。需要将二维数组中的数据传入到QCPGraph中。
3. 设置折线图的样式。可以设置折线的颜色、线型、线宽等属性。
4. 设置坐标轴的样式。可以设置坐标轴的标签、刻度、范围等属性。
5. 将折线图保存为图片或PDF格式。
以下是一个简单的示例代码,可以生成一个包含随机数据的折线图:
```c++
#include <iostream>
#include <fstream>
#include <cmath>
#include "qcustomplot.h"
using namespace std;
int main()
{
// 读取CSV文件并存储数据到二维数组中
double data[100][2];
ifstream file("data.csv");
for (int i = 0; i < 100; i++) {
file >> data[i][0] >> data[i][1];
}
// 创建QCustomPlot对象
QCustomPlot *customPlot = new QCustomPlot();
// 添加一个折线图
QCPGraph *graph = customPlot->addGraph();
// 设置折线图的样式
graph->setPen(QPen(Qt::red));
graph->setLineStyle(QCPGraph::lsLine);
graph->setScatterStyle(QCPScatterStyle::ssDisc);
// 将数据传入到折线图中
QVector<double> x(100), y(100);
for (int i = 0; i < 100; i++) {
x[i] = data[i][0];
y[i] = data[i][1];
}
graph->setData(x, y);
// 设置坐标轴的样式
customPlot->xAxis->setLabel("X Axis");
customPlot->yAxis->setLabel("Y Axis");
// 显示图形
customPlot->replot();
// 将折线图保存为PNG格式图片
customPlot->savePng("lineChart.png");
return 0;
}
```
注意:以上示例代码需要在Qt环境下编译运行。如果您没有安装Qt,可以先下载安装Qt Creator,然后创建一个空的Qt控制台项目,将以上代码复制到main.cpp文件中,然后添加QCustomPlot库的头文件和库文件,最后编译运行即可。
阅读全文
相关推荐
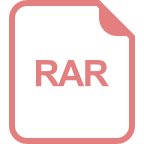
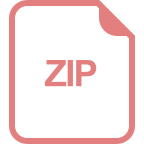
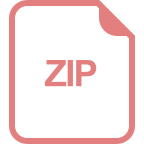
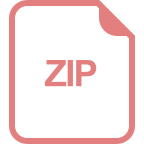
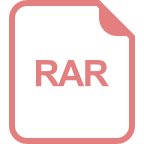
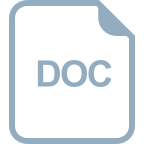
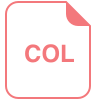
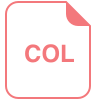
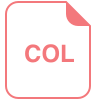
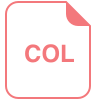
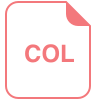
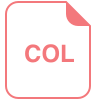
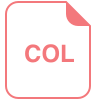
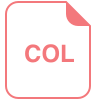
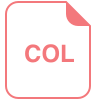