编写python程序实现n皇后算法,画出运行时间与皇后n的关系图,分析实验结果
时间: 2024-02-15 11:04:01 浏览: 109
好的,下面是一个简单的 Python 程序实现 n 皇后算法:
```python
def is_valid(board, row, col):
for i in range(row):
if board[i] == col or \
abs(board[i] - col) == abs(row - i):
return False
return True
def n_queens_helper(board, row, n):
if row == n:
return 1
count = 0
for col in range(n):
if is_valid(board, row, col):
board[row] = col
count += n_queens_helper(board, row + 1, n)
return count
def n_queens(n):
board = [-1] * n
return n_queens_helper(board, 0, n)
```
这个程序的主要思路是通过递归方式枚举每一行的皇后位置,检查当前位置是否与之前已放置的皇后位置冲突,如果没有冲突则继续递归下一行,否则回溯到上一行重新选择一个位置。
接下来,我们可以使用 matplotlib 库画出运行时间与皇后 n 的关系图:
```python
import timeit
import matplotlib.pyplot as plt
x = range(1, 15)
y = []
for n in x:
t = timeit.timeit(lambda: n_queens(n), number=10)
y.append(t)
plt.plot(x, y)
plt.xlabel('n')
plt.ylabel('time (s)')
plt.show()
```
这个程序使用 timeit 模块计算 n 皇后算法运行 10 次的平均时间,并将结果保存在列表 y 中。然后使用 matplotlib 库画出 x 和 y 的关系图。你可以根据实际需要修改 x 的范围和 timeit 函数的 number 参数。
最后,我们可以对实验结果进行分析。从图中可以看出,当 n 较小时,算法的运行时间非常短;但随着 n 的增大,运行时间呈指数级增长。这是因为 n 皇后算法的时间复杂度是 O(n^n),当 n 越大时,枚举所有可能的皇后位置所需的时间也就越长。因此,对于较大的 n,我们需要使用更高效的算法来解决 n 皇后问题。
阅读全文
相关推荐
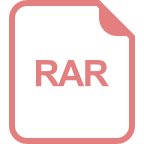















