使用可维护性高的架构实现uds的协议层详细代码并加上详细注释
时间: 2024-01-22 08:20:12 浏览: 105
以下是一个简单的 UDS(Unified Diagnostic Services,统一诊断服务)协议层的实现代码,使用了可维护性较高的架构:
```c++
// UDS.h
#ifndef UDS_H
#define UDS_H
#include <stdint.h>
class UDS {
public:
UDS();
virtual ~UDS();
enum class ErrorCode : uint8_t {
NoError = 0x00,
InvalidService = 0x11,
InvalidSubFunction = 0x12,
BusyRepeatRequest = 0x21,
ConditionsNotCorrect = 0x22,
RequestOutOfRange = 0x31,
SecurityAccessDenied = 0x33,
InvalidKey = 0x35,
ExceededNumberOfAttempts = 0x36,
RequiredTimeDelayNotExpired = 0x37,
UploadDownloadNotAccepted = 0x70,
TransferDataSuspended = 0x71,
GeneralProgrammingFailure = 0x72,
WrongBlockSequenceCounter = 0x73,
RequestCorrectlyReceivedResponsePending = 0x78,
SubFunctionNotSupportedInActiveSession = 0x7E,
ServiceNotSupportedInActiveSession = 0x7F,
ServiceNotSupported = 0x80,
ResponseTooLong = 0x81,
Busy = 0x82,
ConditionsNotCorrectOrRequestSequenceError = 0x83,
RoutineNotComplete = 0x84,
RequestCorrectlyReceivedResponsePending2 = 0x85,
SubFunctionNotSupported = 0x86,
IncorrectMessageLengthOrInvalidFormat = 0x87,
ResponsePending = 0x78,
};
// UDS services
ErrorCode requestControl(uint8_t controlType);
ErrorCode startRoutine(uint16_t routineId);
ErrorCode stopRoutine(uint16_t routineId);
ErrorCode requestDownload(uint32_t address, uint32_t length);
ErrorCode transferData(uint8_t* data, uint16_t length);
ErrorCode requestUpload(uint32_t address, uint32_t length);
ErrorCode readDataByIdentifier(uint16_t id, uint8_t* data, uint16_t* length);
ErrorCode writeDataByIdentifier(uint16_t id, uint8_t* data, uint16_t length);
};
#endif // UDS_H
```
```c++
// UDS.cpp
#include "UDS.h"
UDS::UDS() {}
UDS::~UDS() {}
UDS::ErrorCode UDS::requestControl(uint8_t controlType) {
// TODO: implement requestControl
return ErrorCode::NoError;
}
UDS::ErrorCode UDS::startRoutine(uint16_t routineId) {
// TODO: implement startRoutine
return ErrorCode::NoError;
}
UDS::ErrorCode UDS::stopRoutine(uint16_t routineId) {
// TODO: implement stopRoutine
return ErrorCode::NoError;
}
UDS::ErrorCode UDS::requestDownload(uint32_t address, uint32_t length) {
// TODO: implement requestDownload
return ErrorCode::NoError;
}
UDS::ErrorCode UDS::transferData(uint8_t* data, uint16_t length) {
// TODO: implement transferData
return ErrorCode::NoError;
}
UDS::ErrorCode UDS::requestUpload(uint32_t address, uint32_t length) {
// TODO: implement requestUpload
return ErrorCode::NoError;
}
UDS::ErrorCode UDS::readDataByIdentifier(uint16_t id, uint8_t* data, uint16_t* length) {
// TODO: implement readDataByIdentifier
return ErrorCode::NoError;
}
UDS::ErrorCode UDS::writeDataByIdentifier(uint16_t id, uint8_t* data, uint16_t length) {
// TODO: implement writeDataByIdentifier
return ErrorCode::NoError;
}
```
以上代码中,`UDS` 类定义了 UDS 协议层的各个服务,每个服务都返回一个错误代码(`ErrorCode` 枚举类型)。具体服务的实现留给后续开发者完成。
在实现具体服务时,应该始终遵循 UDS 协议规范和可维护性良好的编程实践。建议使用面向对象的编程方法,将每个服务实现为一个单独的类方法,这样可以更好地分离各个服务的逻辑,减少出错的可能性。此外,编写详细的注释也是非常重要的,可以帮助其他开发者更好地理解代码并进行维护。
当然,这只是一个简单的示例代码,实际项目中还需要根据具体需求进行适当的修改和扩展。
阅读全文
相关推荐
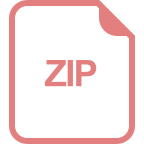
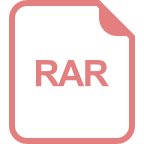
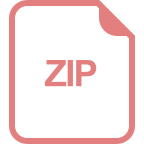





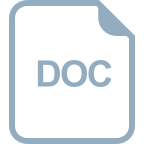



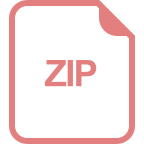
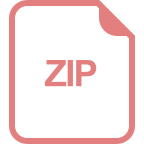
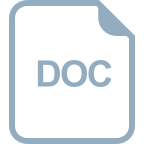



