Write a program to complete the following command: new id - create a specified sequence of Numbers for id (id < 200000) Add id num - to join the sequence of Numbers for id integer num merge id1 id2 - merger sequence number of id1 and id2, and make id2 empty unique id - remove repetitive element in the sequence id out id - from small to large, output the elements of sequence of Numbers for ids separated by Spaces
时间: 2024-03-22 10:41:38 浏览: 83
Sure, I can help you with that! Here's a Python program that implements the commands you've described:
```python
class Sequence:
def __init__(self):
self.sequences = {}
def new(self, id):
if id >= 200000:
print("Error: id should be less than 200000")
return
self.sequences[id] = []
def add(self, id, num):
if id not in self.sequences:
self.new(id)
self.sequences[id].append(num)
def merge(self, id1, id2):
if id1 not in self.sequences or id2 not in self.sequences:
print("Error: invalid ids")
return
self.sequences[id1] += self.sequences[id2]
self.sequences[id2] = []
def unique(self, id):
if id not in self.sequences:
print("Error: invalid id")
return
self.sequences[id] = list(set(self.sequences[id]))
def out(self, id):
if id not in self.sequences:
print("Error: invalid id")
return
sequence = sorted(self.sequences[id])
print(" ".join(str(num) for num in sequence))
sequence = Sequence()
# Example usage:
sequence.new(1)
sequence.add(1, 5)
sequence.add(1, 2)
sequence.add(1, 3)
sequence.add(1, 5)
sequence.unique(1)
sequence.out(1)
sequence.new(2)
sequence.add(2, 6)
sequence.add(2, 1)
sequence.add(2, 4)
sequence.add(2, 3)
sequence.merge(1, 2)
sequence.out(1)
```
Here's an explanation of how it works:
- The `Sequence` class represents a collection of sequences of numbers, identified by an integer ID.
- The `new` method creates a new sequence with the specified ID.
- The `add` method adds a number to the sequence with the specified ID.
- The `merge` method merges two sequences with the specified IDs into one, and empties the second sequence.
- The `unique` method removes any duplicate elements from the sequence with the specified ID.
- The `out` method outputs the elements of the sequence with the specified ID, sorted in ascending order and separated by spaces.
You can test the program by calling the methods on a `Sequence` instance, as shown in the example usage at the end.
阅读全文
相关推荐
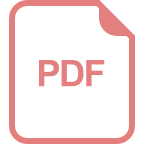
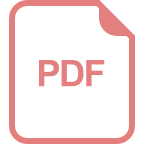
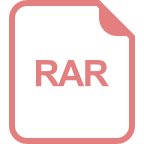



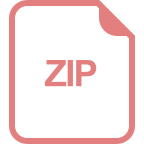
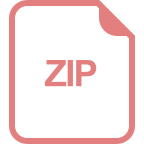
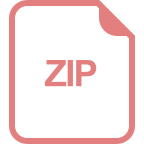
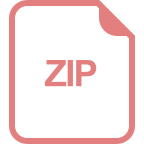
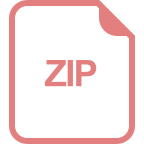
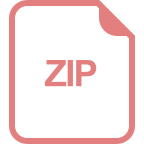
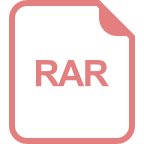