知道一个点的X,Y坐标,根据方位角和长度求距离某点的坐标 c++
时间: 2024-12-22 09:24:25 浏览: 6
当你知道一个点的 X 和 Y 坐标以及另一个点的方向(通常用角度表示,例如北偏东多少度)和长度(距离),可以使用数学中的向量计算来求解新的点的坐标。以下是基于 C++ 的一种简单方法:
首先,你需要将方向转换成弧度,因为大多数数学库函数需要以弧度作为输入。假设起点坐标是 `start_x` 和 `start_y`,终点的方向是 `bearing`(0 表示正北,顺时针增加)和距离 `distance`。
```cpp
#include <cmath> // 包含圆周率 π 和三角函数
// 将角度从度数转换为弧度
double toRadians(double degrees) {
return degrees * M_PI / 180.0;
}
// 计算新点坐标
void calculateNewCoordinates(double start_x, double start_y, double bearing, double distance) {
double end_x = start_x + distance * cos(toRadians(bearing));
double end_y = start_y + distance * sin(toRadians(bearing));
// 返回新的坐标(end_x, end_y)
std::pair<double, double> endPoint = {end_x, end_y};
// 使用std::cout或类似输出机制打印结果
std::cout << "新点坐标: (" << endPoint.first << ", " << endPoint.second << ")" << std::endl;
}
int main() {
double x = 0; // 起点X坐标
double y = 0; // 起点Y坐标
double bearing = 45; // 方位角 (北偏东45度)
double length = 10; // 长度
calculateNewCoordinates(x, y, bearing, length);
return 0;
}
```
阅读全文
相关推荐



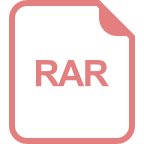













