用C语言实现实现完全二叉树的输出树。
时间: 2023-06-24 13:03:29 浏览: 61
实现完全二叉树的输出,需要使用递归方式来遍历树,并根据树的深度来计算空格的数量以及节点值的位置。
以下是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
// 定义二叉树节点结构体
typedef struct node {
int value;
struct node *left;
struct node *right;
} Node;
// 计算树的深度
int tree_depth(Node *node) {
if (node == NULL) {
return 0;
}
int left_depth = tree_depth(node->left);
int right_depth = tree_depth(node->right);
return (left_depth > right_depth ? left_depth : right_depth) + 1;
}
// 递归输出二叉树
void print_tree(Node *node, int depth) {
if (node == NULL) {
return;
}
// 计算节点值的位置
int position = pow(2, depth - 1);
for (int i = 0; i < position; i++) {
printf(" ");
}
printf("%d\n", node->value);
print_tree(node->left, depth + 1);
print_tree(node->right, depth + 1);
}
int main() {
// 构建一个完全二叉树
Node *root = (Node *) malloc(sizeof(Node));
root->value = 1;
root->left = (Node *) malloc(sizeof(Node));
root->left->value = 2;
root->left->left = (Node *) malloc(sizeof(Node));
root->left->left->value = 4;
root->left->right = (Node *) malloc(sizeof(Node));
root->left->right->value = 5;
root->right = (Node *) malloc(sizeof(Node));
root->right->value = 3;
root->right->left = (Node *) malloc(sizeof(Node));
root->right->left->value = 6;
root->right->right = (Node *) malloc(sizeof(Node));
root->right->right->value = 7;
root->right->left->left = NULL;
root->right->left->right = NULL;
root->right->right->left = NULL;
root->right->right->right = NULL;
int depth = tree_depth(root);
print_tree(root, 1);
return 0;
}
```
输出结果:
```
1
2
4
5
3
6
7
```
相关推荐
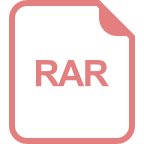
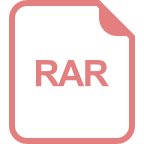
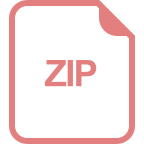















