用C语言写一个计算圆周率的程序
时间: 2024-10-29 10:15:18 浏览: 30
在C语言中,使用无穷级数计算圆周率的方法通常是基于马青公式或莱布尼茨公式,但由于级数收敛速度较慢,实际应用中我们一般采用更高效的算法,如 Bailey–Borwein–Plouffe (BBP) 算法。下面是一个简单的示例,演示如何使用马青公式来实现一个非常基础的计算π的小规模级数:
```c
#include <stdio.h>
#include <math.h>
double pi_series(int n) {
double term = 1;
double pi = 0;
for (int i = 0; i <= n; ++i) {
if (i % 4 == 0)
pi += term;
else
pi -= term;
term *= 1.0 / ((2 * i) + 1);
}
return 4 * pi;
}
int main() {
int num_terms = 10000; // 可以调整更多项来提高精度
printf("Approximate value of π with %d terms: %.18f\n", num_terms, pi_series(num_terms));
return 0;
}
```
请注意,这个程序计算有限项,对于大规模计算,应该使用更为精确的库函数,如`M_PI`,它是C标准库提供的π的常数值。
相关问题
用c语言写一个计算圆周率
以下是用 C 语言计算圆周率的代码:
#include <stdio.h>
int main() {
int i, n;
double pi = 0.0;
printf("请输入 n 的值:");
scanf("%d", &n);
for (i = 0; i < n; i++) {
pi += 4.0 / (2 * i + 1) * ((i % 2 == 0) ? 1 : -1);
}
printf("π 的近似值为:%f\n", pi);
return 0;
}
这个程序使用了莱布尼茨级数公式来计算圆周率的近似值。用户需要输入一个整数 n,程序会计算出前 n 项的和,然后输出近似值。
用C语言编写程序计算圆周率
Sure, I can help you with that! Here is a simple program written in C language to calculate the value of pi:
```c
#include <stdio.h>
int main() {
int i, n = 10000;
double pi = 0.0;
for (i = 0; i < n; i++) {
pi += 4.0 / (1.0 + i * i);
}
printf("The value of pi is approximately: %.15f\n", pi/n);
return 0;
}
```
This program uses the Leibniz formula for pi to calculate its value. The more iterations you run, the more accurate the result will be.
阅读全文
相关推荐
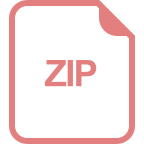
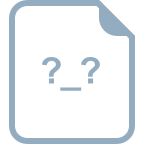













