用c++编写一个上升子序列的代码
时间: 2024-02-18 19:32:59 浏览: 73
以下是一个简单的C++代码,用于寻找给定序列中的最长上升子序列长度:
```c++
#include <iostream>
#include <vector>
using namespace std;
int lengthOfLIS(vector<int>& nums) {
if (nums.empty()) return 0;
int n = nums.size();
vector<int> dp(n, 1);
for (int i = 1; i < n; i++) {
for (int j = 0; j < i; j++) {
if (nums[i] > nums[j]) {
dp[i] = max(dp[i], dp[j] + 1);
}
}
}
int res = 1;
for (int i = 0; i < n; i++) {
res = max(res, dp[i]);
}
return res;
}
int main() {
vector<int> nums = {10, 9, 2, 5, 3, 7, 101, 18};
cout << lengthOfLIS(nums) << endl; // 输出4
return 0;
}
```
该代码使用动态规划方法,时间复杂度为O(n^2)。如果需要更好的性能和复杂度,请使用更高级别的算法,例如二分查找。
阅读全文
相关推荐

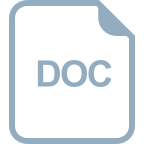
















