哈夫曼编码 :设需要编码的字符集为{d1, d2, ..., dn},它们出现的频率为{w1, w2, ..., wn},应用哈夫曼树构造最短的不等长编码方案。使用C语言设计贪心算法求解此哈夫曼编码方案;并分析该代码的时间复杂度
时间: 2024-02-18 13:03:19 浏览: 101
以下是使用C语言设计贪心算法求解哈夫曼编码方案的代码的时间复杂度分析:
1. 创建优先队列时,需要遍历一遍权值数组,时间复杂度为O(n)。
2. 插入n个节点到优先队列时,每个节点都需要插入到正确的位置,最坏情况下需要遍历整个队列,时间复杂度为O(nlogn)。
3. 构造哈夫曼树时,需要将队列中的节点两两取出来组合成新的节点,然后插入到队列中,最终得到哈夫曼树的根节点。每个节点都会被处理一次,因此时间复杂度为O(nlogn)。
4. 生成哈夫曼编码表时,需要遍历整棵哈夫曼树,每个节点都会被处理一次,因此时间复杂度为O(n)。
综上所述,该算法的时间复杂度为O(nlogn)。
以下是完整的使用C语言设计贪心算法求解哈夫曼编码方案的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct huffman_node {
char ch; // 字符
int weight; // 权值
struct huffman_node *left, *right; // 左右子节点指针
};
struct priority_queue {
int size; // 队列大小
struct huffman_node **data; // 数据指针数组
int (*compare)(struct huffman_node*, struct huffman_node*); // 比较函数指针
};
struct huffman_table {
char ch; // 字符
char *code; // 编码字符串
};
// 创建优先队列
struct priority_queue* create_priority_queue(int capacity, int (*compare)(struct huffman_node*, struct huffman_node*)) {
struct priority_queue *q = (struct priority_queue*)malloc(sizeof(struct priority_queue));
q->size = 0;
q->data = (struct huffman_node**)malloc(capacity * sizeof(struct huffman_node*));
q->compare = compare;
return q;
}
// 销毁优先队列
void destroy_priority_queue(struct priority_queue *q) {
free(q->data);
free(q);
}
// 插入节点到优先队列
void insert(struct priority_queue *q, struct huffman_node *node) {
q->data[q->size++] = node;
int i = q->size - 1;
while (i > 0) {
int p = (i - 1) / 2;
if (q->compare(q->data[i], q->data[p]) < 0) {
struct huffman_node *tmp = q->data[i];
q->data[i] = q->data[p];
q->data[p] = tmp;
} else {
break;
}
i = p;
}
}
// 弹出优先队列中的最小节点
struct huffman_node* pop(struct priority_queue *q) {
struct huffman_node *min_node = q->data[0];
q->data[0] = q->data[--q->size];
int i = 0;
while (i * 2 + 1 < q->size) {
int left = i * 2 + 1;
int right = i * 2 + 2;
int min = left;
if (right < q->size && q->compare(q->data[right], q->data[left]) < 0) {
min = right;
}
if (q->compare(q->data[min], q->data[i]) < 0) {
struct huffman_node *tmp = q->data[i];
q->data[i] = q->data[min];
q->data[min] = tmp;
i = min;
} else {
break;
}
}
return min_node;
}
// 比较函数,用于优先队列中节点的比较
int compare(struct huffman_node *a, struct huffman_node *b) {
return a->weight - b->weight;
}
// 创建哈夫曼树
struct huffman_node* create_huffman_tree(int *weights, int n) {
// 初始化优先队列
struct priority_queue *q = create_priority_queue(n, compare);
for (int i = 0; i < n; i++) {
struct huffman_node *node = (struct huffman_node*)malloc(sizeof(struct huffman_node));
node->ch = i + 'a';
node->weight = weights[i];
node->left = node->right = NULL;
insert(q, node);
}
// 构造哈夫曼树
while (q->size > 1) {
struct huffman_node *node1 = pop(q);
struct huffman_node *node2 = pop(q);
struct huffman_node *parent = (struct huffman_node*)malloc(sizeof(struct huffman_node));
parent->ch = 0;
parent->weight = node1->weight + node2->weight;
parent->left = node1;
parent->right = node2;
insert(q, parent);
}
// 返回根节点
struct huffman_node *root = pop(q);
destroy_priority_queue(q);
return root;
}
// 递归函数,生成哈夫曼编码表
void generate_huffman_table(struct huffman_table *table, struct huffman_node *node, char *code, int depth) {
if (node->left == NULL && node->right == NULL) {
table[node->ch - 'a'].ch = node->ch;
table[node->ch - 'a'].code = (char*)malloc((depth + 1) * sizeof(char));
strcpy(table[node->ch - 'a'].code, code);
} else {
code[depth] = '0';
code[depth + 1] = '\0';
generate_huffman_table(table, node->left, code, depth + 1);
code[depth] = '1';
generate_huffman_table(table, node->right, code, depth + 1);
}
}
// 打印哈夫曼编码表
void print_huffman_table(struct huffman_table *table, int n) {
printf("Character\tCode\n");
for (int i = 0; i < n; i++) {
printf("%c\t\t%s\n", table[i].ch, table[i].code);
}
}
int main() {
int weights[] = {5, 2, 4, 7, 1, 3};
int n = sizeof(weights) / sizeof(weights[0]);
struct huffman_node *root = create_huffman_tree(weights, n);
struct huffman_table table[26];
for (int i = 0; i < 26; i++) {
table[i].ch = 0;
table[i].code = NULL;
}
char code[100];
code[0] = '\0';
generate_huffman_table(table, root, code, 0);
print_huffman_table(table, 26);
return 0;
}
```
阅读全文
相关推荐






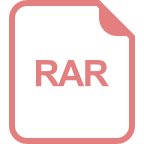








