用c语言写一个小游戏可以玩的俄罗斯方块的代码
时间: 2023-06-06 13:06:18 浏览: 117
好的,我可以为您提供一个C语言编写的小游戏俄罗斯方块的代码。注意,此代码仅供学习参考,同时不保证完全无问题。
首先,您需要定义一些变量,例如:
```c
int board[20][10] = {0}; // 存储方块的位置状态
int block[7][4][4] = { // 存储方块的形状
{ {0,0,0,0}, {1,1,1,1}, {0,0,0,0}, {0,0,0,0} },
{ {0,0,1,0}, {0,0,1,0}, {0,0,1,0}, {0,0,1,0} },
{ {0,0,0,0}, {0,1,1,0}, {0,1,1,0}, {0,0,0,0} },
{ {0,0,0,0}, {0,1,0,0}, {0,1,1,1}, {0,0,0,0} },
{ {0,0,0,0}, {0,0,1,1}, {0,1,1,0}, {0,0,0,0} },
{ {0,0,0,0}, {0,1,1,0}, {0,0,1,1}, {0,0,0,0} },
{ {0,0,0,0}, {0,0,0,0}, {1,1,1,1}, {0,0,0,0} }
};
int colors[8] = { // 方块颜色
0x000000, 0xff0000, 0x00ff00, 0x0000ff,
0xffff00, 0xff00ff, 0x00ffff, 0x888888
};
int cur_block, cur_x, cur_y, cur_rot; // 当前方块、位置、旋转状态
```
接下来,您需要实现一些函数来处理方块的移动、旋转和碰撞检测等操作,例如:
```c
// 方块移动
void block_move(int dx, int dy) {
if (!block_collision(cur_block, cur_x + dx, cur_y + dy, cur_rot)) {
cur_x += dx;
cur_y += dy;
}
}
// 方块旋转
void block_rotate(int dr) {
int new_rot = (cur_rot + dr + 4) % 4;
if (!block_collision(cur_block, cur_x, cur_y, new_rot)) {
cur_rot = new_rot;
}
}
// 碰撞检测
int block_collision(int b, int x, int y, int r) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (block[b][i][j]) {
int px = x + j;
int py = y + i;
if (px < 0 || px >= 10 || py < 0 || py >= 20 || board[py][px]) {
return 1;
}
}
}
}
return 0;
}
```
最后,您需要主程序来完成游戏逻辑,例如:
```c
int main() {
// 初始化游戏
cur_block = rand() % 7;
cur_x = 4;
cur_y = 0;
cur_rot = 0;
// 游戏循环
while (1) {
// 显示方块
for (int i = 0; i < 20; i++) {
for (int j = 0; j < 10; j++) {
draw_block(j * 30, i * 30, colors[board[i][j]]);
}
}
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (block[cur_block][i][j]) {
draw_block((cur_x + j) * 30, (cur_y + i) * 30, colors[cur_block + 1]);
}
}
}
// 按键检测
if (key_down(VK_LEFT)) {
block_move(-1, 0);
}
if (key_down(VK_RIGHT)) {
block_move(1, 0);
}
if (key_down(VK_DOWN)) {
block_move(0, 1);
}
if (key_down(VK_SPACE)) {
while (!block_collision(cur_block, cur_x, cur_y + 1, cur_rot)) {
cur_y++;
}
}
if (key_down(VK_UP)) {
block_rotate(1);
}
// 方块下落
if (frame_count % 20 == 0) {
if (block_collision(cur_block, cur_x, cur_y + 1, cur_rot)) {
// 方块着陆
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (block[cur_block][i][j]) {
board[cur_y + i][cur_x + j] = cur_block + 1;
}
}
}
// 消行处理
for (int i = 0; i < 20; i++) {
int full = 1;
for (int j = 0; j < 10; j++) {
if (board[i][j] == 0) {
full = 0;
}
}
if (full) {
for (int j = i; j > 0; j--) {
for (int k = 0; k < 10; k++) {
board[j][k] = board[j - 1][k];
}
}
for (int j = 0; j < 10; j++) {
board[0][j] = 0;
}
}
}
// 新方块生成
cur_block = rand() % 7;
cur_x = 4;
cur_y = 0;
cur_rot = 0;
if (block_collision(cur_block, cur_x, cur_y, cur_rot)) {
break; // 游戏结束
}
} else {
// 方块下落
block_move(0, 1);
}
}
// 刷新屏幕
refresh_screen();
// 帧数更新
frame_count++;
}
return 0;
}
```
好了,以上就是C语言编写的小游戏俄罗斯方块的代码。祝您学习愉快!
阅读全文
相关推荐
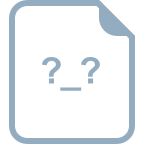

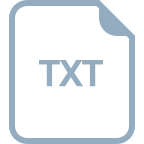
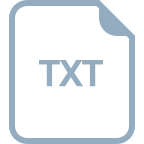
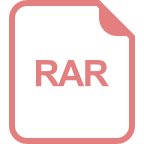
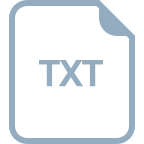









