用c写一个线索二叉树中序遍历算法
时间: 2024-04-29 22:26:45 浏览: 13
好的,我可以回答这个问题。以下是用C语言编写线索二叉树的中序遍历算法:
```c
// 定义线索二叉树的结点
typedef struct ThreadedBinaryTreeNode {
int data; // 数据域
struct ThreadedBinaryTreeNode *leftChild; // 左孩子指针
struct ThreadedBinaryTreeNode *rightChild; // 右孩子指针
int ltag; // 左线索标志位
int rtag; // 右线索标志位
} TBNode;
// 初始化线索二叉树
void InitThreadedBinaryTree(TBNode *root) {
root->leftChild = root;
root->rightChild = root;
root->ltag = 0;
root->rtag = 1;
}
// 在中序遍历的过程中线索化二叉树
TBNode* InThread(TBNode *p, TBNode *pre) {
if (p != NULL) {
// 中序遍历左子树
pre = InThread(p->leftChild, pre);
// 处理当前结点
if (p->leftChild == NULL) {
p->leftChild = pre;
p->ltag = 1;
}
if (pre != NULL && pre->rightChild == NULL) {
pre->rightChild = p;
pre->rtag = 1;
}
pre = p;
// 中序遍历右子树
pre = InThread(p->rightChild, pre);
}
return pre;
}
// 建立线索二叉树
void CreateThreadedBinaryTree(TBNode *root) {
if (root != NULL) {
TBNode *pre = NULL;
// 中序遍历线索化二叉树
pre = InThread(root, pre);
// 处理最后一个结点的线索
pre->rightChild = root;
pre->rtag = 1;
root->rightChild = pre;
}
}
// 中序遍历线索二叉树
void InOrderThreadedBinaryTree(TBNode *root) {
TBNode *p = root->leftChild;
while (p != root) {
// 找到中序遍历的起点
while (p->ltag == 0) {
p = p->leftChild;
}
// 输出当前结点
printf("%d ", p->data);
// 跳到其后继结点
while (p->rtag == 1 && p->rightChild != root) {
p = p->rightChild;
printf("%d ", p->data);
}
// 转向右子树的根
p = p->rightChild;
}
}
```
Hope it helps!
相关推荐
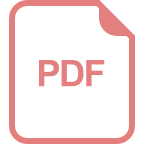
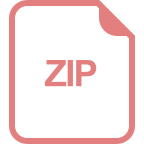














